Upgrading to Workspace
We will be discontinuing the Eikon Desktop soon in favour of our next generation data and analytics workflow solution, LSEG Workspace. This page is designed to help you assess any changes you may need to make to programmatic (API) workflows. We also provide resources here to help you make those changes as well.
Upgrading to Workspace
Other related resources
Eikon 4 Desktop API (dotNet) Upgrade:
Symbology
Symbology API
When working with symbol conversion, the majority of use cases involves the ability to request for a 1:1 mapping from a symbol, in one of many common formats, to a best match of another. For example convert an ISIN into a RIC. However, symbol conversion can extend into the requirement of converting to all available matches. That is, depending on the conversion, there may be a 1:many mapping. For example, convert an ISIN into all available RICs for the given ISIN, as opposed to the best match.
In this section, we'll outline the approach used by both libraries.
Eikon .Net APIs
What does 'IDataServices.ReferenceData' provide?
This service provides the ability to request for 1 or more symbols of the same type to be converted to another type. The supported types using this interface are ISIN, SEDOL, CUSIP, Ticker and RIC. If the conversion involves a 1:many relationship, the result set will provide the entire list of converted results for the specific input symbol requested. In addition, the response will also include a "best match", which is a common requirement when performing basic symbology mapping.
To demonstrate a simple request for a best match of both an ISIN and CUSIP to be mapped to a RIC, the following can be used:
// Converting ISIN to RIC - best match
services.ReferenceData.RequestSymbols("US5949181045", SymbolType.Isin, SymbolsBestMatchResponse);
// Converting CUSIP to RIC - best match
services.ReferenceData.RequestSymbols("037833100", SymbolType.Cusip, SymbolsBestMatchResponse);
private void SymbolsBestMatchResponse(SymbolsResponse response)
{
if (response.HasError)
{
Console.WriteLine(response.Error.Message);
}
else
{
var request = response.RequestedSymbols.ToArray();
var result = response.Symbols.ToArray();
for (int i = 0; i < response.Count; i++)
Console.WriteLine($"{request[i]} => {result[i].ErrorMessage ?? result[i].BestMatch.Ric}");
}
}
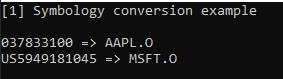
Extending the above example, we can take one of the conversions and instead provide a mapping of a symbol to all available RICs. The following code simply pulls out the result collection as opposed to the best match:
// Converting ISIN to RIC - Top 25
services.ReferenceData.RequestSymbols("US0378331005", SymbolType.Isin, SymbolsAllResponse);
private void SymbolsAllResponse(SymbolsResponse response)
{
if (response.HasError)
{
Console.WriteLine(response.Error.Message);
}
else
{
var request = response.RequestedSymbols.ToArray();
var result = response.Symbols.ToArray();
var cnt = 25;
for (int i = 0; i < response.Count; i++)
{
Console.WriteLine($"{request[i]} => {result[i].ErrorMessage ?? $"{cnt} results"}");
foreach (var value in result[i].Rics.Take(cnt))
Console.WriteLine($"\t{value}");
}
}
}
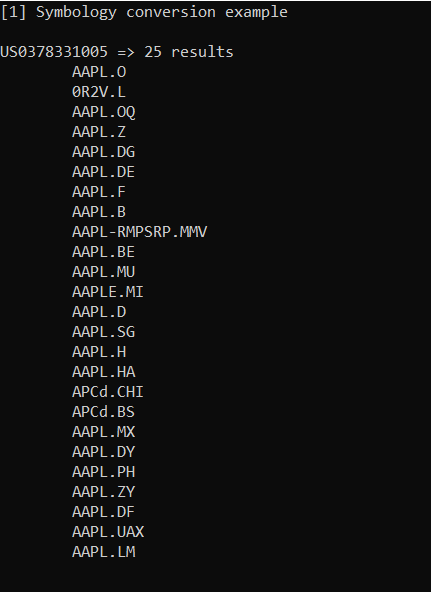
Data Library for .Net
The Data Library for .Net provides an equivalent service allowing developers to request for symbol mappings that include both a "best match" as well as the equivalent 1:many conversion results. However, the Data Library for .Net uses separate interfaces to achieve the 2 distinct capabilities.
The following interfaces are supported:
- Data.Content.Symbology.SymbolConversion
Provides a simple best match conversion supporting the ability to specify a mixed set of symbols to be converted to common symbols such as ISIN, SEDOL, CUSIP, LipperID, PermID, RIC and Ticker.
- Data.Content.SearchService.Search
A powerful search mechanism that includes the ability to pull out a 1:many mapping but also allows metadata to be pulled down and includes powerful filtering mechanisms to narrow down result sets.
What does 'Data.Content.Symbology.SymbolConversion' provide?
The SymbolConversion interface provides the ability to specify 1 or more symbols, of any type, to be mapped to 1 or more symbols using its "best match" algorithm. The service automatically includes a description of the symbol for display/reference purposes. As with the Eikon .Net API, the service also allows the explicit specification of 'from' symbol type, which can be used for validation purposes.
To demonstrate a simple request for a best match of both an ISIN and CUSIP to be mapped to a RIC, the following can be used:
// Mixed symbols (ISIN, CUSIP) to RIC conversion - best match
var response = SymbolConversion.Definition().Symbols("US5949181045", "037833100")
.ToSymbolType(SymbolConversion.SymbolType.RIC)
.GetData();
Common.DisplayTable("Mixed symbols (ISIN, CUSIP) to RIC conversion - best match:", response);

What does 'Data.Content.Search.SearchService' provide?
The Search interface provides access to a powerful API accessing a wealth of content covering content such as quotes, instruments, organizations, and many other assets that can be programmatically integrated within your business Workflow. As part of its capabilities, the service can be used to provide symbol conversion queries allowing for 1:many mapping results using various symbol types. In addition, we also have the ability to pull down much more than the basic description and symbol mappings including metadata that may be useful describing the details of the mapping. Because we are using the Search service, the filtering and details extracted can be endless.
It may be worthwhile to familiarize yourself with this powerful service as outline within this article: Building Search into your Application Workflow.
To demonstrate a request to provide all mappings for an ISIN to RIC conversion:
// ISIN to RIC conversion - Top 25
// Note: Only show those results where the asset is 'AC' (Active)
var response = Search.Definition().Filter("IssueISIN eq 'US0378331005 and AssetState eq 'AC'")
.Select("DocumentTitle, RIC")
.Top(25)
.GetData();
Common.DisplayTable("ISIN to RIC conversion - top 25:", response);
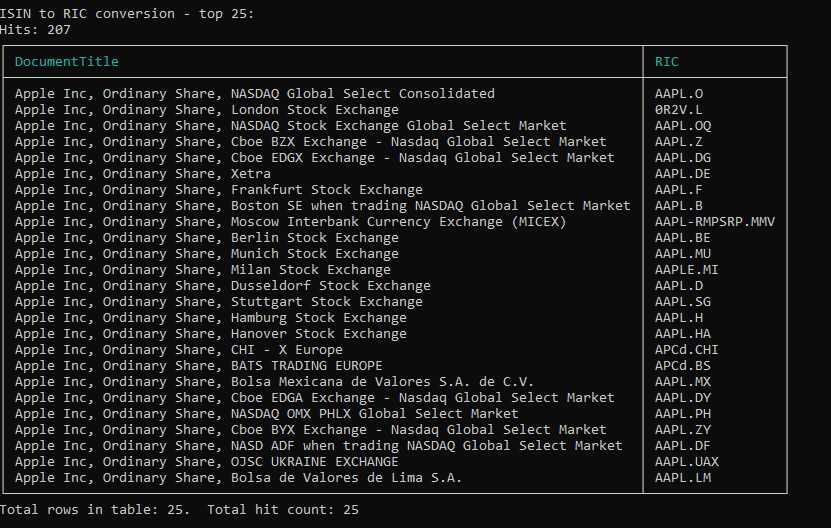
The above request contains a filter expression explicitly searching for all RICs associated with the ISIN value. The filter expression offers quite a bit of power when choosing the collection of hits and offers total control over the ability to filter unwanted results by expanding the expression. Because of the power offered within the service, users can easily extend the capability to cover additional symbol mapping expressions. For example, we could have instead chose to return all RICs associated with other popular symbol mapping requirements. For example:
Mapping | Filter Expression |
---|---|
CUSIP | "CUSIP eq '037833100' and AssetState eq 'AC'" |
SEDOL | "SedolSecondaryQuoteValue eq 'BH4HKS3 and AssetState eq 'AC'' |
TICKER | ""TickerSymbol xeq 'IBM' and AssetState eq 'AC'" |
So long as the data is available, we can build powerful expressions to pull out very specific data mappings. In addition, choose alternative output fields beyond the simple description of the match.
Conclusion
Moving from your Eikon .Net API to the Data Library for .Net should provide you with a path to acquire the desired content within your applications. As we continue to improve and expand our content set, we anticipate there may be some differences. The goal of this article is to provide solutions that not only meet your needs but hopefully include additional value not present with the legacy platform. Please report any issues or challenges within our Q&A site using the tag: workspace-upgrade and we'll continue to update this article to do our best to ensure we can provide a suitable migration path.