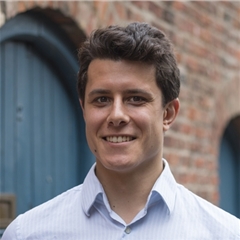
In this article, we build the Python function Get_IBES_GA with ipywidgets Dropdowns to retrieve Institutional Brokers' Estimate System (IBES) Global Aggregate earnings data for country and regional sectors in an interactive way. With this function in CodeBook, no need to know how to code, it's as simple as click and play!
This is only Part 1; I will attempt to see the best ways in which the bellow can be used to extract insights, possibly in graphical ways. If you have a workflow in mind for which the above would be useful, I would be happy to hear about it. Please do not hesitate to submit your propositions to jonathan.legrand@refinitiv.com
Returns a Pandas data-frame with:
- PE Ratio
- Earnings Growth
- PEG Ratio
- EPS
- Risk Premium (data not available for Regions)
- EV/EBITDA
- Revision Ratio
- Dividend Yield
Overview _Fiscal_Years
- Ratios are displayed only for Fiscal Years (FY0, FY1, FY2, FY3)
- PE Ratio
- Earnings Growth
- PEG Ratio
- EPS
- Risk Premium (data not available for Regions, only for Countries)
- EV/EBITDA
- Revision Ratio
- Dividend Yield
Development Tools & Resources
The example code demonstrating the use case is based on the following development tools and resources:
- Refinitiv's DataStream Web Services (DSWS): Access to DataStream data. A DataStream or Refinitiv Workspace IDentification (ID) will be needed to run the code below.
Get to Coding
We need to gather our data. Since Refinitiv's DataStream Web Services (DSWS) allows for access to ESG data covering nearly 70% of global market cap and over 400 metrics, naturally it is more than appropriate. We can access DSWS via the Python library "DatastreamDSWS" that can be installed simply by using pip install.
import DatastreamDSWS as dsws
# We can use our Refinitiv's Datastream Web Socket (DSWS) API keys that allows us to be identified by Refinitiv's back-end services and enables us to request (and fetch) data: Credentials are placed in a text file so that it may be used in this code without showing it itself.
(dsws_username, dsws_password) = (open("Datastream_username.txt","r"),
open("Datastream_password.txt","r"))
ds = dsws.Datastream(username = str(dsws_username.read()),
password = str(dsws_password.read()))
# It is best to close the files we opened in order to make sure that we don't stop any other services/programs from accessing them if they need to.
dsws_username.close()
dsws_password.close()
# # Alternatively one can use the following:
# import getpass
# dsusername = input()
# dspassword = getpass.getpass()
# ds = dsws.Datastream(username = dsusername, password = dspassword)
import warnings # ' warnings ' is a native Python library allowing us to raise warnings and errors to users.
from datetime import datetime # This is needed to keep track of when code runs.
import pandas as pd # pandas is an open source data analysis and manipulation tool that allows us to use data-frames. Version 1.1.4 was used in this article.
import numpy as np # NumPy is a library allowing for array manipulations including linear algebra, fourier transform, and matrices. Version 1.20.1 was used in this article.
from tqdm.notebook import trange # ' tqdm ' allows loops to show a progress meter. Version 4.48.2 was used in this article.
import ipywidgets as widgets # ipywidgets is a library of interactive HTML widgets for Jupyter notebooks and the IPython kernel. Version 7.5.1 was used in this article.
from IPython.display import display # This allows us to display data-frames.
for i,j in zip([pd, np, tqdm, widgets],["pandas", "numpy", "tqdm", "ipywidgets"]):
print(f"The library {j} imported is version {i.__version__}")
The library pandas imported is version 1.1.4
The library numpy imported is version 1.20.1
The library tqdm imported is version 4.48.2
The library ipywidgets imported is version 7.5.1
Step by Step Example
Collecting Datastream Data
Users ought to be able to select their Country/Region of interest without knowing the Datastream Mnemonics for them. In line with this, the bellow creates tables of reference for Countries/Regions and such Mnemonics and Python functions to allow users easy data retrieval.
Data-Frame of Countries, Regions and their Complimentary DSWS Mnemonic and IBES Code
# # From the ' Reference data.xls ' file, one could run and use the following:
# xl_countries = pd.read_excel("Reference data.xls", sheet_name = "Countries")
# xl_regions = pd.read_excel("Reference data.xls", sheet_name = "Regions")
# xl_index = pd.read_excel("Reference data.xls", sheet_name = "Index Names 3")
# xl_columns = pd.read_excel("Reference data.xls", sheet_name = "Column Names 2")
# # If you don't have the ' Reference data.xls ' file, just use the bellow:
xl_regions = pd.DataFrame(data = {'Region': {0: 'EAFE', 1: 'EAFE + Canada',2: ... '@:M1WLDXA'}})
xl_countries = pd.DataFrame(data = {'Region': {0: 'Argentina',1: 'Australia',2: ... ,50: '@:VEMSCIP'}})
# We will use the following to index and column our final data-frame on interest:
xl_index = pd.DataFrame(data = {'Category': {0: 'Energy', 1: 'Energy', 2: 'Energy', ... 88: 'M3MU', 89: 'M3WU'}})
xl_columns = pd.DataFrame({'Column 1': {0: 'PE-Ratio', 1: 'PE-Ratio', 2: 'PE-Ratio', ... 31: '18M', 32: '', 33: ''}})
# # Merging the two data-frames together:
xl_regions = xl_regions.append(xl_countries, ignore_index = True)
Python function Region_to_DSWS_region_mnemonic_and_IBES_code
As per its written definition bellow, this function returns a string of the Datastream Web Service (DSWS) region's mnemonic and IBES code. For certain requests, DSWS needs a ticker specified with a nomenclature that includes DSWS region's mnemonic.
def Region_to_DSWS_region_mnemonic_and_IBES_code(region = None, xl_regions = None):
"""Region_to_DSWS_region_mnemonic_and_IBES_code Version 1.0:
This function returns a string of the Datastream Web Service (DSWS) region's mnemonic and IBES code.
DSWS is Refinitiv's API retrieving data from Datastream to a Python Pandas data-frame. For information on DSWS, please visit 'https://developers.refinitiv.com/en/api-catalog/eikon/datastream-web-service'.
For certain requests, DSWS needs a ticker specified with a nomenclature that includes DSWS region's mnemonic. For an example, see 'Examples' bellow.
Parameters:
----------
region: str
Region of choice's name (e.g.: 'EAFE-ex-UK').
It has to be one of the elements in the following list: ['EAFE' 'EAFE + Canada' ... 'USA' 'Venezuela']
Default: region = None
xl_regions: Pandas data-frame
The data-frame of regions to map with, including columns 'Region', 'Mnemonic' and 'Code'.
This function (' Region_to_DSWS_region_mnemonic_and_IBES_code ') will map ' region ' to 'Mnemonic' and 'Code' in this data-frame and return them.
If ' None ', a pre-defined data-frame is used.
It is named with 'xl' at its start because it originally came from the Excel workbook 'Datastream IBES Global Aggregates MSCI.xlsm'.
Default: xl_regions = None
Dependencies:
----------
pandas 1.0.3
Examples:
--------
>>> Region_to_DSWS_region_mnemonic_and_IBES_code("EAFE + Canada")
('FC', '@:M1EAFEC')
"""
if xl_regions == None: xl_regions = pd.DataFrame(data = {'Region': {0: 'EAFE', 1: 'EAFE + Canada', ... , 81: '@:VEMSCIP'}})
if region not in xl_regions["Region"].to_list():
print("Invalid ' region ' argument specified.")
else:
return xl_regions[xl_regions["Region"] == region]["Mnemonic"].values[0], xl_regions[xl_regions["Region"] == region]["Code"].values[0]
For example, we could get the Mnemonic and Code for the United Kingdom:
Region_to_DSWS_region_mnemonic_and_IBES_code("United Kingdom")
('UK', '@:UKMSCIP')
ordered_mnemonic = "M1E1,M2E2, ... ,M3WU"
# The long string for ' IBESGA_fields ' (and subsequently ' IBESGA_tickers_str ') can be found in the ' Datastream IBES Global Aggregates MSCI .xlsm ' file.
IBESGA_fields = "ALNAME,AF0PE, ... ,ADVYLD"
IBESGA_fields = IBESGA_fields.split(",")
# Calling it with ' _str ' at the end to dissociate it from other objects and use it later:
IBESGA_tickers_str = "M1CD,M1CS, ... ,M3WU"
IBESGA_full_tickers = ["@:" + Region_to_DSWS_region_mnemonic_and_IBES_code("EAFE + Canada")[0] + i for i in IBESGA_tickers_str.split(",")]
Now we can go ahead and collect our data from Datastream. Note that we split requests in batches using ds_get_twice_data to keep well within request limits.
# Defined our dsws data retrieval function
def ds_get_twice_data(tickers, fields, batch = 15, kind = 0):
df = ds.get_data(tickers = tickers[0],
fields = fields[:batch],
kind = kind)
_df = ds.get_data(tickers = tickers[0],
fields = fields[batch:],
kind = kind)
df = df.append(_df, ignore_index = True)
for i in tickers[1:]:
_df1 = ds.get_data(tickers = i,
fields = fields[:batch],
kind = kind)
_df2 = ds.get_data(tickers = i,
fields = fields[batch:],
kind = kind)
_df = _df1.append(_df2, ignore_index = True)
df = df.append(_df, ignore_index = True)
return df
# Collect our data info data-frame ' df ':
df = ds_get_twice_data(tickers = IBESGA_full_tickers, fields = IBESGA_fields, batch = 15, kind = 0)
# Tidy our ' df ' and replace stings 'NA' with computationally recognisable nan values:
df = pd.DataFrame(
index = df["Instrument"].unique(), columns = df["Datatype"].unique(),
data = [list(df["Value"][df["Instrument"] == i]) for i in df["Instrument"].unique()]).replace('NA', np.nan, regex=True)
# Let's keep the tiker names:
df["Tickers"] = df.index
df.index = IBESGA_tickers_str.split(",")
df = df.T[ordered_mnemonic.split(",")].T
# Now re-index the data-frame:
_index = [list(xl_index.fillna(method='ffill').loc[i].values) for i in range(len(xl_index.index))]
df.index = pd.MultiIndex.from_tuples(_index)
# # re-column the data-frame:
# We need to add a column named 'ALNAME'. Since it's a column with 3 levels, it needs to be added thrice.
xl_columns2 = pd.concat([pd.DataFrame({'Column 1': {0: 'ALNAME'}, 'Column 2': {0: ''}, 'Column 3': {0: ''}}), xl_columns], ignore_index = True)
# We need to add a column named 'Tickers' similarlly.
xl_columns2 = pd.concat([xl_columns2, pd.DataFrame({'Column 1': {0: 'Tickers'}, 'Column 2': {0: ''}, 'Column 3': {0: ''}})], ignore_index = True)
# Collumns 'PEG-Ratio' 'Fiscal Year', 'FY0' to 'FY3' happen to be calculated, not pulled from DSWS, so we need to ignore them now and add them later
xl_columns3 = xl_columns2.drop([11,12,13,14]).reset_index(drop = True)
_columns = [list(xl_columns3.fillna(method='ffill').loc[i].values) for i in range(len(xl_columns3.index))]
df.columns = pd.MultiIndex.from_tuples(_columns)
# PEG-Ratio columns need to be calculated:
for i in range(4):
df["PEG-Ratio", "Fiscal Year", f"FY{i}"] = df["PE-Ratio", "Fiscal Year", f"FY{i}"] / df["Earnings Growth", "Fiscal Year", f"FY{i}"]
Now let's see our data-frame:
|
ALNAME | PE-Ratio | Earnings Growth | ... | EV/EBITDA | Rev. Ratio | Div Yield | Tickers | PEG-Ratio | |||||||||||||||
Fiscal Year | Forward | Fiscal Year | ... | Fiscal Year | Forward | Fiscal Year | ||||||||||||||||||
FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | ... | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | ||||||||
Energy | Energy | Energy | M1E1 | MSCI EAFE + Canada Energy Sector | 79.201 | 12.338 | 10.531 | 9.848 | 11.675 | 10.801 | -85.188 | 541.945 | 17.161 | ... | 4.571 | 5.127 | 4.853 | 5.3305 | 4.71 | @:FCM1E1 | -0.92972 | 0.022766 | 0.613659 | 1.42127 |
M2E2 | MSCI EAFE + Canada Energy Industry Group | 79.201 | 12.338 | 10.531 | 9.848 | 11.675 | 10.801 | -85.188 | 541.945 | 17.161 | ... | 4.571 | 5.127 | 4.853 | 5.3305 | 4.71 | @:FCM2E2 | -0.92972 | 0.022766 | 0.613659 | 1.42127 | |||
Energy Equipment & Services | M3ES | MSCI EAFE + Canada Energy Equipment & Services... | NaN | 36.341 | 28.034 | 21.719 | 33.074 | 29.144 | -101.587 | NaN | 29.634 | ... | 8.057 | 10.783 | 10.033 | 18.1818 | 1.931 | @:FCM3ES | NaN | NaN | 0.946008 | 0.746922 | ||
Oil, Gas & Consumable Fuels | M3OG | MSCI EAFE + Canada Oil, Gas & Consumable Fuels... | 78.641 | 12.284 | 10.487 | 9.813 | 11.625 | 10.756 | -85.114 | 540.201 | 17.133 | ... | 4.563 | 5.116 | 4.843 | 5.0218 | 4.729 | @:FCM3OG | -0.92395 | 0.02274 | 0.612094 | 1.42755 | ||
Materials | Materials | Materials | M1M1 | MSCI EAFE + Canada Materials Sector | 22.863 | 13.408 | 13.971 | 14.576 | 13.694 | 14.111 | 6.28 | 70.514 | -4.029 | ... | 6.928 | 6.592 | 6.806 | 13.9673 | 2.787 | @:FCM1M1 | 3.64061 | 0.190147 | -3.46761 | -3.3655 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
Utilities | Utilities | Utilities | M2U2 | MSCI EAFE + Canada Utilities Industry Group | 18.152 | 17.298 | 16.033 | 15.189 | 16.982 | 16.334 | 4.348 | 4.937 | 7.891 | ... | 9.043 | 9.721 | 9.717 | 1.9969 | 4.015 | @:FCM2U2 | 4.17479 | 3.50375 | 2.03181 | 2.73233 |
Electric Utilities | M3EU | MSCI EAFE + Canada Electric Utilities Industry | 17.176 | 17.407 | 16.315 | 15.333 | 17.118 | 16.574 | 7.758 | -1.324 | 6.693 | ... | 8.806 | 9.989 | 10 | 0 | 3.871 | @:FCM3EU | 2.21397 | -13.1473 | 2.43762 | 2.39541 | ||
Gas Utilities | M3GU | MSCI EAFE + Canada Gas Utilities Industry | 19.641 | 18.267 | 16.938 | 15.918 | 17.94 | 17.292 | 3.493 | 7.518 | 7.851 | ... | 8.916 | 9.711 | 9.549 | 7.619 | 4.23 | @:FCM3GU | 5.62296 | 2.42977 | 2.15743 | 2.48408 | ||
Multi-Utilities | M3MU | MSCI EAFE + Canada Multi-Utilities Industry | 19.432 | 15.926 | 14.41 | 13.819 | 15.55 | 14.736 | 0.387 | 22.015 | 10.527 | ... | 8.914 | 8.776 | 8.863 | 2.0833 | 4.235 | @:FCM3MU | 50.2119 | 0.723416 | 1.36886 | 3.2363 | ||
Water Utilities | M3WU | MSCI EAFE + Canada Water Utilities Industry | 20.794 | 19.466 | 17.276 | 18.123 | 19.263 | 18.126 | -23.781 | 6.82 | 12.675 | ... | 12.921 | 13.206 | 12.859 | 13.3333 | 4.328 | @:FCM3WU | -0.8744 | 2.85425 | 1.363 | -3.87741 |
90 rows × 36 columns
We can select any section of our data-frame for focused analysis
df["PEG-Ratio"]
|
Fiscal Year | ||||||
FY0 | FY1 | FY2 | FY3 | ||||
Energy | Energy | Energy | M1E1 | -0.92972 | 0.022766 | 0.613659 | 1.42127 |
M2E2 | -0.92972 | 0.022766 | 0.613659 | 1.42127 | |||
Energy Equipment & Services | M3ES | NaN | NaN | 0.946008 | 0.746922 | ||
Oil, Gas & Consumable Fuels | M3OG | -0.92395 | 0.02274 | 0.612094 | 1.42755 | ||
Materials | Materials | Materials | M1M1 | 3.64061 | 0.190147 | -3.46761 | -3.3655 |
... | ... | ... | ... | ... | ... | ... | ... |
Utilities | Utilities | Utilities | M2U2 | 4.17479 | 3.50375 | 2.03181 | 2.73233 |
Electric Utilities | M3EU | 2.21397 | -13.1473 | 2.43762 | 2.39541 | ||
Gas Utilities | M3GU | 5.62296 | 2.42977 | 2.15743 | 2.48408 | ||
Multi-Utilities | M3MU | 50.2119 | 0.723416 | 1.36886 | 3.2363 | ||
Water Utilities | M3WU | -0.8744 | 2.85425 | 1.363 | -3.87741 |
90 rows × 4 columns
Create the Python function Get_IBES_GA with ipywidgets Dropdowns
# Create a list to append with our returned data-frame:
DSWS_IBES_GA = []
# Define our drop down specificities:
drop_down_IBES_GA_return = widgets.Dropdown(
options = [""] + xl_regions["Region"].to_list(),
value = "",
disabled = False)
# Define our drop down specificities for predefined variables:
drop_down_predefined_variables = widgets.Dropdown(
options = [""] + ["Yes", "No"],
value = "",
description = "Use predefined ordered_mnemonic, IBESGA_fields, xl_index and xl_columns2 variables?",
disabled = False)
# Create the function to programmatically return the data-frame of interest
def Get_IBES_GA(area, # ' area ' example: "EAFE + Canada"
loading_bar = True,
display_df = True,
append_DSWS_IBES_GA = True,
ordered_mnemonic = "predefined",
IBESGA_fields = "predefined",
xl_index = "predefined",
xl_columns2 = "predefined",
max_row_col = True,
export_to_excel = True):
"""Get_IBES_GA Version 1.0:
If ' display_df ' is set to True, this function returns a dataframe of Datastream Web Service (DSWS) data of Institutional Brokers' Estimate System (IBES) Global Aggregate earnings for country and regional sectors in an interactive way.
If ' append_DSWS_IBES_GA ' is set to True, user need to have defined a Python list ' DSWS_IBES_GA ', it will then be appended.
DSWS is Refinitiv's API retrieving data from Datastream to a Python Pandas data-frame. For information on DSWS, please visit 'https://developers.refinitiv.com/en/api-catalog/eikon/datastream-web-service'.
Parameters:
----------
area: str
Region of choice's name (e.g.: 'EAFE-ex-UK' or 'EAFE + Canada').
It has to be one of the elements in the following list: ['EAFE' 'EAFE + Canada' 'EAFE-ex-UK' 'EASEA (EAFE-ex-Japan)' 'EM (Emerging Markets)' 'EM Asia' 'EM Eastern Europe' 'EM Europe' 'EM Europe + Middle East' 'EM Europe, Middle East & Africa' 'EM Far East' 'EM Latin America' 'EMU (Euro)' 'EMU (US Dollar)' 'EMU + UK' 'Europe' 'Europe-ex-EMU' 'Europe-ex-UK' 'Far East' 'G7 Index' 'Kokusai (World-ex-Japan)' 'Nordic Countries' 'North America' 'Pacific' 'Pacific-ex-Japan' 'World' 'World-ex-Australia' 'World-ex-EMU' 'World-ex-Europe' 'World-ex-UK' 'World-ex-USA' 'Argentina' 'Australia' 'Australia' 'Austria' 'Belgium' 'Brazil' 'Canada' 'Chile' 'China' 'Colombia' 'Czech Republic' 'Denmark' 'Egypt' 'Finland' 'France' 'Germany' 'Greece' 'Hong Kong' 'Hungary' 'India' 'Indonesia' 'Ireland' 'Israel' 'Italy' 'Japan' 'Jordan' 'Korea' 'Malaysia' 'Mexico' 'Morocco' 'Netherlands' 'New Zealand' 'Norway' 'Pakistan' 'Peru' 'Philippines' 'Poland' 'Portugal' 'Russia' 'Singapore' 'South Africa' 'Spain' 'Sri Lanka' 'Sweden' 'Switzerland' 'Taiwan' 'Thailand' 'Turkey' 'United Kingdom' 'USA' 'Venezuela']
It has no default values, but as per the Example bellow, you may want to use this function in conjuncture with ' ipywidgets.interact '.
loading_bar: Boolean
If set to True, then a loading bar will appear, keeping track of the dsws requests made - the steps that take longest in this function.
Default: loading_bar = True
display_df: Boolean
If set to True, the resulting data-frame will be displayed.
Default: display_df = True
append_DSWS_IBES_GA: Boolean
If set to True, user needs to have pre-created an empty Python lisy named ' DSWS_IBES_GA ' which will be populated with the data-frame returned.
Default: append_DSWS_IBES_GA = True
ordered_mnemonic: str or Pandas data-frame
If set to "predefined", then a predefined Pandas data-frame of mnemonics is used.
User may enter his/her own Pandas data-frame, but (s)he will need to change all of the following parameters accordingly: ordered_mnemonic, IBESGA_fields, xl_index, and xl_columns2.
Default: ordered_mnemonic = "predefined"
IBESGA_fields: str or Pandas data-frame
If set to "predefined", then a predefined Pandas data-frame of Datastream IBESGA fields is used.
User may enter his/her own Pandas data-frame, but (s)he will need to change all of the following parameters accordingly: ordered_mnemonic, IBESGA_fields, xl_index, and xl_columns2.
Default: IBESGA_fields = "predefined"
xl_index: str or Pandas data-frame
If set to "predefined", then a predefined Pandas data-frame is used.
User may enter his/her own Pandas data-frame, but (s)he will need to change all of the following parameters accordingly: ordered_mnemonic, IBESGA_fields, xl_index, and xl_columns2.
Default: xl_index = "predefined"
xl_columns2: str or Pandas data-frame
If set to "predefined", then a predefined Pandas data-frame is used.
User may enter his/her own Pandas data-frame, but (s)he will need to change all of the following parameters accordingly: ordered_mnemonic, IBESGA_fields, xl_index, and xl_columns2.
Default: xl_columns2 = "predefined"
max_row_col: Boolean
If set to True, all of the resulted data-frame's columns and indices (rows) will be displayed.
Default: max_row_col = True
export_to_excel: Boolean
If se to True, an excel workbook with one sheet of the returned data-frame will be generated where the python file is run.
Default: True
Dependencies:
----------
pandas 1.1.4 as pd
numpy 1.20.1 as np
tqdm 4.48.2 via ' from tqdm.notebook import trange '
DatastreamDSWS as dsws
warnings
Optional:
ipywidgets 7.5.1
Examples:
----------
>>> import DatastreamDSWS as dsws
>>> ds = dsws.Datastream(username = "insert dsws username here", password = "insert dsws password here")
>>> from datetime import datetime
>>> import warnings
>>> from datetime import date
>>> import pandas as pd
>>> import numpy as np
>>> import ipywidgets as widgets
>>> from IPython.display import display
>>>
>>> xl_regions = pd.DataFrame(data = {'Region': {0: 'EAFE', 1: 'EAFE + Canada',2: 'EAFE-ex-UK', ... ,30: '@:M1WLDXA'}})
>>> xl_countries = pd.DataFrame(data = {'Region': {0: 'Argentina',1: 'Australia', ... ,50: '@:VEMSCIP'}})
>>> xl_regions = xl_regions.append(xl_countries, ignore_index = True)
>>>
>>> DSWS_IBES_GA = []
>>>
>>> drop_down_IBES_GA_return = widgets.Dropdown(options = [""] + xl_regions["Region"].to_list(),value = "", disabled = False)
>>>
>>> drop_down_predefined_variables = widgets.Dropdown(options = [""] + ["Yes", "No"], value = "", description = "Use predefined ordered_mnemonic, IBESGA_fields, xl_index and xl_columns2 variables?", disabled = False)
>>>
>>> def Get_IBES_GA_predefined(area):
>>> _df = Get_IBES_GA(area)
>>> display(_df)
>>>
>>> def IBES_GA_predefined_variables(predefined):
>>> if predefined == "Yes":
>>> widgets.interact(Get_IBES_GA_predefined, area = drop_down_IBES_GA_return);
>>> elif predefined == "No":
>>> widgets.interact(Get_IBES_GA, area = drop_down_IBES_GA_return);
>>>
>>> widgets.interact(IBES_GA_predefined_variables, predefined = drop_down_predefined_variables);
>>>
>>> # display(DSWS_IBES_GA[0])
"""
# If the ' area ' is chosen in the following dropdown as an empty value (i.e.: ""), then we don't want to return anything:
if area == "":
pass
else:
# Set data-frame display conditions:
if max_row_col == True:
pd.set_option('display.max_row', None)
pd.set_option('display.max_columns', None)
# # Define reference Python objects:
if ordered_mnemonic == "predefined": ordered_mnemonic = "M1E1,M2E2 ... ,M3MU,M3WU"
else: ordered_mnemonic = ordered_mnemonic
# The long string for ' IBESGA_fields ' (and subsequently ' IBESGA_tickers_str ') can be found in the ' Datastream IBES Global Aggregates MSCI .xlsm ' file.
if IBESGA_fields == "predefined":
IBESGA_fields = "ALNAME,AF0PE, ... ,ADVYLD"
IBESGA_fields = IBESGA_fields.split(",")
# Calling it with ' _str ' at the end to dissociate it from other objects and use it later.
IBESGA_tickers_str = "M1CD,M1CS, ... ,M3WU"
# # Define the list of tickers to pull data for from DSWS
def Region_to_DSWS_region_mnemonic_and_IBES_code(region = None):
"""For description of this function, see 'How to collect Datastream IBES Global Aggregate Earnings Data' article on the Refinitiv/LSEG Developer Community Article Catalogue."""
xl_regions = pd.DataFrame(data = {'Region': {0: 'EAFE', 1: 'EAFE + Canada' ... , 81: '@:VEMSCIP'}})
if region not in xl_regions["Region"].to_list():
warnings.warn("Invalid ' region ' argument specified.")
else:
return xl_regions[xl_regions["Region"] == region]["Mnemonic"].values[0], xl_regions[xl_regions["Region"] == region]["Code"].values[0]
IBESGA_full_tickers = ["@:" + Region_to_DSWS_region_mnemonic_and_IBES_code(area)[0] + i for i in IBESGA_tickers_str.split(",")]
else:
IBESGA_full_tickers = IBESGA_fields
# # Get coding:
# Create 1st data-frame to subsequentially append
df0 = ds.get_data(tickers = IBESGA_full_tickers[0],
fields = IBESGA_fields[:15],
kind = 0)
df1 = df0.append(ds.get_data(tickers = IBESGA_full_tickers[0],
fields = IBESGA_fields[15:],
kind = 0),
ignore_index = True)
## Append our data-frame with each ticker
# Leave the option for a loading bar:
if loading_bar == True: J = trange(len(IBESGA_full_tickers), # colour = '#001EFF',
desc = 'requests', write_bytes = True)
else: J = range(len(IBESGA_full_tickers[1:]))
# Now request data:
for i,j in zip(IBESGA_full_tickers, J):
if i == IBESGA_full_tickers[0]:
pass
else:
# Create a placeholder data-frame ' _df ' to append onto our previously defined data-frame ' df1 '
_df1 = ds.get_data(tickers = i,
fields = IBESGA_fields[:15],
kind = 0)
_df2 = ds.get_data(tickers = i,
fields = IBESGA_fields[15:],
kind = 0)
_df = _df1.append(_df2, ignore_index = True)
df1 = df1.append(_df, ignore_index = True)
# Rearrange our data-frame to have tickers as index and fields as columns
df2 = pd.DataFrame(
index = df1["Instrument"].unique(), columns = df1["Datatype"].unique(),
data = [list(df1["Value"][df1["Instrument"] == i]) for i in df1["Instrument"].unique()])
# Replace str 'NA' with numpy nan values
df2 = df2.replace(['NA'], np.nan)
for i in range(4):
df2[f"PEGRatio{i}"] = df2[f"AF{i}PE"] / df2[f"AF{i}GRO"]
# Need to Rearrange the columns in order
df2 = df2.reindex(df2.columns[1:11].tolist() + [f"PEGRatio{i}" for i in range(4)] + df2.columns[11:-4].tolist(), axis=1)
# We don't want to loose the data of which row is for which ticker with the next few lines, so let's create a column with that information
df2["Tickers"] = df2.index
# Rename our data-frame's index to fit a standard easier to work with
df2.index = IBESGA_tickers_str.split(",")
# Rearrange the order of the rows
df3 = df2.T[ordered_mnemonic.split(",")].T
# We will use the following ' xl_index ' data-frame to index our returned data-frame
if xl_index == "predefined":
xl_index = pd.DataFrame(data = {'Category': {0: 'Energy', 1: 'Energy', ... , 89: 'M3WU'}})
else:
xl_index = xl_index
# Now re-index the data-frame:
_columns = [list(xl_index.fillna(method='ffill').loc[i].values) for i in range(len(xl_index.index))]
df3.index = pd.MultiIndex.from_tuples(_columns)
## Now we need to rename the columns:
# If you have the accompanying excel workbook, you can run: ' xl_columns2 = pd.read_excel("Reference data.xls", sheet_name = "Column Names 2").fillna(method='ffill') ''
if xl_columns2 == "predefined":
xl_columns2 = pd.DataFrame(data = {'Column 1': {0: 'PE-Ratio', 1: 'PE-Ratio', ... 34: 'Tickers'}})
else:
xl_columns2 = xl_columns2
_columns = [list(xl_columns2.loc[i].values) for i in range(len(xl_columns2.index))]
df3.columns = pd.MultiIndex.from_tuples(_columns)
# Append out previously defined list with the dataframe created
try:
if append_DSWS_IBES_GA == True: DSWS_IBES_GA.append(df3)
except:
warnings.warn("If ' append_DSWS_IBES_GA ' is set to True, user needs to define an empty Python list ' append_DSWS_IBES_GA ' before running ' Get_IBES_GA '.")
# Export to excel sheet if asked:
if export_to_excel == True:
df3.to_excel(excel_writer = f"DSWS_IBES_GA_{area}.xlsx",
sheet_name = datetime.now().strftime("%Y.%m.%d_%Hh.%Mm"))
# Just to check if this function worked expectedly, let's return the number of rows in the outputed data-frame
if display_df == True: return df3
Let's setup and use an interactive widget:
def Get_IBES_GA_predefined(area):
_df = Get_IBES_GA(area)
display(_df)
def IBES_GA_predefined_variables(predefined):
if predefined == "Yes":
widgets.interact(Get_IBES_GA_predefined, area = drop_down_IBES_GA_return);
elif predefined == "No":
widgets.interact(Get_IBES_GA, area = drop_down_IBES_GA_return);
widgets.interact(IBES_GA_predefined_variables, predefined = drop_down_predefined_variables);
From there one can interact with widgets:
You may hover over concatinated text to read it all
And you may select from dropdowns
More interestingly, you may select the area of interest
Then, data is collected as the loading bar fills up before rendering our results
|
PE-Ratio | Earnings Growth | PEG-Ratio | EPS Mean | Risk premium | EV/EBITDA | Rev. Ratio | Div Yield | Tickers | |||||||||||||||||||||||||||||
Fiscal Year | Forward | Fiscal Year | Fiscal Year | Fiscal Year | Forward | Fiscal Year | Forward | Fiscal Year | Forward | Rev. Ratio | Div Yield | Tickers | ||||||||||||||||||||||||||
FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | FY0 | FY1 | FY2 | FY3 | FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | 12M | 18M | Rev. Ratio | Div Yield | Tickers | ||||
Energy | Energy | Energy | M1E1 | 460.023 | 16.258 | 13.12 | 12.335 | 15.046 | 13.549 | -96.896 | 2729.53 | 23.914 | 6.369 | -4.7476 | 0.005956 | 0.548633 | 1.93672 | 0.336 | 9.515 | 11.791 | 12.542 | 10.282 | 11.418 | NaN | NaN | NaN | NaN | NaN | NaN | 9.515 | 6.273 | 5.54 | 5.25 | 6.011 | 5.652 | 15.0922 | 4.567 | @:KKM1E1 |
M2E2 | 460.023 | 16.258 | 13.12 | 12.335 | 15.046 | 13.549 | -96.896 | 2729.53 | 23.914 | 6.369 | -4.7476 | 0.005956 | 0.548633 | 1.93672 | 0.336 | 9.515 | 11.791 | 12.542 | 10.282 | 11.418 | NaN | NaN | NaN | NaN | NaN | NaN | 9.515 | 6.273 | 5.54 | 5.25 | 6.011 | 5.652 | 15.0922 | 4.567 | @:KKM2E2 | |||
Energy Equipment & Services | M3ES | 48.738 | 24.336 | 16.978 | 12.999 | 21.264 | 17.879 | -61.793 | 100.27 | 43.339 | 30.608 | -0.78873 | 0.242705 | 0.391749 | 0.424693 | 1.914 | 3.834 | 5.495 | 7.177 | 4.387 | 5.218 | NaN | NaN | NaN | NaN | NaN | NaN | 10.221 | 9.821 | 8.184 | 6.571 | 9.214 | 8.422 | 60 | 1.933 | @:KKM3ES | ||
Oil, Gas & Consumable Fuels | M3OG | 754.708 | 16.012 | 12.984 | 12.306 | 14.845 | 13.399 | -98.128 | 4613.39 | 23.323 | 5.512 | -7.69106 | 0.003471 | 0.556704 | 2.23258 | 0.216 | 10.202 | 12.582 | 13.275 | 11.004 | 12.192 | NaN | NaN | NaN | NaN | NaN | NaN | 9.485 | 6.171 | 5.458 | 5.204 | 5.917 | 5.567 | 10.2171 | 4.692 | @:KKM3OG | ||
Materials | Materials | Materials | M1M1 | 24.337 | 15.256 | 15.8 | 16.168 | 15.466 | 15.853 | -0.406 | 59.526 | -3.441 | -2.37 | -59.9433 | 0.256291 | -4.59169 | -6.82194 | 16.089 | 25.665 | 24.782 | 24.218 | 25.316 | 24.699 | NaN | NaN | NaN | NaN | NaN | NaN | 9.202 | 7.408 | 7.765 | 7.937 | 7.556 | 7.769 | 18.7947 | 2.466 | @:KKM1M1 |
M2M2 | 24.337 | 15.256 | 15.8 | 16.168 | 15.466 | 15.853 | -0.406 | 59.526 | -3.441 | -2.37 | -59.9433 | 0.256291 | -4.59169 | -6.82194 | 16.089 | 25.665 | 24.782 | 24.218 | 25.316 | 24.699 | NaN | NaN | NaN | NaN | NaN | NaN | 9.202 | 7.408 | 7.765 | 7.937 | 7.556 | 7.769 | 18.7947 | 2.466 | @:KKM2M2 | |||
Chemicals | M3CH | 30.134 | 22.245 | 20.789 | 19.053 | 21.712 | 21.001 | -11.668 | 35.463 | 7.007 | 9.111 | -2.58262 | 0.627273 | 2.96689 | 2.09121 | 16.695 | 22.616 | 24.2 | 26.405 | 23.171 | 23.956 | NaN | NaN | NaN | NaN | NaN | NaN | 13.859 | 12.249 | 11.695 | 11.045 | 12.05 | 11.776 | 23.3813 | 1.995 | @:KKM3CH | ||
Construction Materials | M3CM | 20.782 | 17.937 | 16.01 | 14.567 | 17.245 | 16.302 | -3.319 | 15.858 | 12.038 | 9.904 | -6.26152 | 1.1311 | 1.32996 | 1.47082 | 13.536 | 15.683 | 17.571 | 19.311 | 16.312 | 17.256 | NaN | NaN | NaN | NaN | NaN | NaN | 8.454 | 9.033 | 8.225 | 7.689 | 8.752 | 8.353 | 26.6055 | 2.156 | @:KKM3CM | ||
Containers & Packaging | M3CT | 21.836 | 18.241 | 16.117 | 14.806 | 17.247 | 16.267 | -9.876 | 19.711 | 13.18 | 7.342 | -2.21102 | 0.925422 | 1.22284 | 2.01662 | 16.238 | 19.439 | 22.001 | 23.949 | 20.56 | 21.798 | NaN | NaN | NaN | NaN | NaN | NaN | 10.344 | 10.207 | 9.158 | 8.866 | 9.755 | 9.254 | 16.6667 | 2 | @:KKM3CT | ||
Metals & Mining | M3MM | 20.305 | 10.422 | 11.899 | 13.816 | 10.942 | 11.869 | 15.7 | 94.834 | -12.412 | -13.877 | 1.29331 | 0.109897 | -0.95867 | -0.9956 | 20.513 | 39.966 | 35.006 | 30.148 | 38.068 | 35.092 | NaN | NaN | NaN | NaN | NaN | NaN | 6.593 | 4.943 | 5.55 | 6.002 | 5.173 | 5.521 | 7.6225 | 3.217 | @:KKM3MM | ||
Paper & Forest Products | M3PF | 21.168 | 14.034 | 16.437 | 16.372 | 14.753 | 15.981 | -4.005 | 50.832 | -14.619 | 0.397 | -5.28539 | 0.276086 | -1.12436 | 41.2393 | 9.832 | 14.829 | 12.661 | 12.711 | 14.106 | 13.023 | NaN | NaN | NaN | NaN | NaN | NaN | 10.491 | 8.528 | 9.35 | 9.269 | 8.784 | 9.201 | 50 | 2.498 | @:KKM3PF | ||
Industrials | Industrials | Industrials | M1ID | 38.012 | 25.669 | 21.344 | 18.795 | 23.949 | 21.891 | -32.919 | 48.085 | 20.323 | 13.077 | -1.15471 | 0.533826 | 1.05024 | 1.43726 | 9.494 | 14.059 | 16.908 | 19.201 | 15.069 | 16.485 | NaN | NaN | NaN | NaN | NaN | NaN | 15.635 | 14.22 | 12.389 | 11.402 | 13.514 | 12.63 | 23.5447 | 1.629 | @:KKM1ID |
Capital Goods | Capital Goods | M2CG | 35.588 | 24.677 | 20.62 | 18.26 | 23.051 | 21.114 | -35.761 | 44.217 | 19.677 | 13.021 | -0.99516 | 0.558089 | 1.04792 | 1.40235 | 9.65 | 13.917 | 16.656 | 18.808 | 14.899 | 16.266 | NaN | NaN | NaN | NaN | NaN | NaN | 14.874 | 14.13 | 12.239 | 11.201 | 13.395 | 12.476 | 22.0871 | 1.699 | @:KKM2CG | |
Aerospace & Defense | M3AD | 60.095 | 25.637 | 19.173 | 16.342 | 23.01 | 19.987 | -67.659 | 134.406 | 33.715 | 17.321 | -0.8882 | 0.190743 | 0.568679 | 0.943479 | 8.239 | 19.312 | 25.823 | 30.295 | 21.517 | 24.771 | NaN | NaN | NaN | NaN | NaN | NaN | 22.278 | 15.34 | 12.396 | 10.799 | 14.215 | 12.799 | 6.5527 | 1.45 | @:KKM3AD | ||
Building Products | M3BP | 27.988 | 23.267 | 20.783 | 18.933 | 22.249 | 21.052 | -4.169 | 20.292 | 11.95 | 9.772 | -6.71336 | 1.14661 | 1.73916 | 1.93747 | 12.157 | 14.624 | 16.371 | 17.971 | 15.292 | 16.162 | NaN | NaN | NaN | NaN | NaN | NaN | 12.955 | 13.177 | 12.122 | 11.324 | 12.766 | 12.254 | 33.7121 | 1.412 | @:KKM3BP | ||
Construction & Engineering | M3CN | 29.554 | 20.199 | 15.902 | 14.193 | 18.53 | 16.486 | -44.204 | 46.317 | 27.02 | 12.038 | -0.66858 | 0.436103 | 0.588527 | 1.17902 | 16.039 | 23.468 | 29.809 | 33.398 | 25.582 | 28.753 | NaN | NaN | NaN | NaN | NaN | NaN | 8.124 | 8.025 | 6.953 | 7.127 | 7.641 | 7.116 | 1.7544 | 2.972 | @:KKM3CN | ||
Electronic Equipment & Instruments | M3EI | 34.149 | 26.911 | 24.233 | 21.679 | 25.82 | 24.509 | -6.623 | 26.896 | 11.049 | 9.805 | -5.15612 | 1.00056 | 2.19323 | 2.21101 | 3.505 | 4.448 | 4.94 | 5.521 | 4.636 | 4.884 | NaN | NaN | NaN | NaN | NaN | NaN | 15.629 | 15.039 | 13.917 | 12.775 | 14.612 | 14.035 | 28.8136 | 1.196 | @:KKM3EI | ||
Industrial Conglomerates | M3IC | 30.95 | 22.84 | 19.637 | 17.362 | 21.517 | 19.979 | -33.445 | 35.507 | 16.311 | 13.54 | -0.9254 | 0.643253 | 1.20391 | 1.28227 | 4.73 | 6.409 | 7.455 | 8.432 | 6.804 | 7.328 | NaN | NaN | NaN | NaN | NaN | NaN | 11.825 | 14.08 | 12.15 | 10.731 | 13.265 | 12.345 | 25.7485 | 1.836 | @:KKM3IC | ||
Machinery | M3MC | 32.562 | 24.616 | 21.305 | 19.227 | 23.285 | 21.699 | -22.564 | 32.28 | 15.542 | 10.806 | -1.4431 | 0.762577 | 1.3708 | 1.77929 | 21.943 | 29.026 | 33.537 | 37.161 | 30.685 | 32.928 | NaN | NaN | NaN | NaN | NaN | NaN | 15.024 | 14.263 | 12.787 | 12.251 | 13.689 | 12.969 | 31.4332 | 1.683 | @:KKM3MC | ||
Trading Companies & Distributors Industry | M3TC | 25.016 | 22.991 | 20.446 | 18.214 | 22.165 | 20.911 | -1.684 | 8.81 | 12.449 | 12.25 | -14.8551 | 2.60965 | 1.64238 | 1.48686 | 20.242 | 22.025 | 24.767 | 27.801 | 22.846 | 24.216 | NaN | NaN | NaN | NaN | NaN | NaN | 10.657 | 11.443 | 10.381 | 9.289 | 11.086 | 10.558 | 13.3333 | 1.738 | @:KKM3TC | ||
Commercial Services & Supplies | Commercial Services & Supplies | M2C2 | 33.587 | 29.412 | 26.122 | 22.809 | 28.183 | 26.573 | -3.289 | 14.194 | 12.597 | 12.324 | -10.2119 | 2.07214 | 2.07367 | 1.85078 | 8.029 | 9.169 | 10.324 | 11.823 | 9.569 | 10.149 | NaN | NaN | NaN | NaN | NaN | NaN | 17.198 | 16.23 | 14.534 | 13.315 | 15.649 | 14.811 | 25.1641 | 1.464 | @:KKM2C2 | |
Commercial Services & Supplies | M3C3 | 35.924 | 31.59 | 28.387 | 24.785 | 30.3 | 28.735 | 1.769 | 13.722 | 11.284 | 12.573 | 20.3075 | 2.30214 | 2.51569 | 1.97129 | 6.758 | 7.686 | 8.553 | 9.796 | 8.013 | 8.449 | NaN | NaN | NaN | NaN | NaN | NaN | 15.569 | 15.091 | 13.528 | 12.39 | 14.569 | 13.792 | 17.7305 | 1.336 | @:KKM3C3 | ||
Transportation | Transportation | M2TR | 53.824 | 27.13 | 21.459 | 18.841 | 24.86 | 22.217 | -37.762 | 98.395 | 26.795 | 13.592 | -1.42535 | 0.275725 | 0.800858 | 1.38618 | 9.784 | 19.411 | 24.541 | 27.951 | 21.183 | 23.703 | NaN | NaN | NaN | NaN | NaN | NaN | 17.145 | 13.568 | 11.881 | 11.194 | 12.902 | 12.114 | 26.8634 | 1.493 | @:KKM2TR | |
Air Freight & Couriers | M3AF | 23.481 | 18.544 | 17.43 | 16.622 | 18.362 | 17.807 | 23.379 | 26.624 | 6.391 | 4.861 | 1.00436 | 0.696514 | 2.72727 | 3.41946 | 13.206 | 16.721 | 17.79 | 18.655 | 16.887 | 17.413 | NaN | NaN | NaN | NaN | NaN | NaN | 11.507 | 10.768 | 10.292 | 10.096 | 10.643 | 10.401 | 43.3735 | 1.649 | @:KKM3AF | ||
Airlines | M3AL | NaN | NaN | 20.079 | 9.507 | NaN | 38.894 | -398.184 | NaN | NaN | 90.2 | NaN | NaN | NaN | 0.105399 | -44.106 | -15.7 | 7.046 | 14.88 | -6.955 | 3.637 | NaN | NaN | NaN | NaN | NaN | NaN | -10.761 | 36.019 | 6.961 | 5.249 | 13.894 | 7.918 | -7.2917 | 0.687 | @:KKM3AL | ||
Marine | M3MA | 22.851 | 11.016 | 14.868 | 13.704 | 12.057 | 14.049 | 172.952 | 107.444 | -25.911 | 8.493 | 0.132123 | 0.102528 | -0.57381 | 1.61356 | 21.273 | 44.131 | 32.696 | 35.473 | 40.319 | 34.602 | NaN | NaN | NaN | NaN | NaN | NaN | 26.332 | 5.73 | 7.333 | 6.751 | 6.176 | 7.002 | 31.5789 | 1.928 | @:KKM3MA | ||
Road & Rail | M3RR | 39.396 | 28.293 | 24.028 | 21.123 | 26.807 | 24.654 | 15.396 | 39.242 | 18.475 | 13.755 | 2.55885 | 0.720988 | 1.30057 | 1.53566 | 26.717 | 37.202 | 43.804 | 49.83 | 39.265 | 42.693 | NaN | NaN | NaN | NaN | NaN | NaN | 16.751 | 16.046 | 14.359 | 13.469 | 15.443 | 14.611 | 41.0569 | 1.433 | @:KKM3RR | ||
Transportation Infrastructure | M3TI | NaN | 662.062 | 49.975 | 30.378 | 102.955 | 51.555 | -146.422 | NaN | 1224.8 | 64.51 | NaN | NaN | 0.040803 | 0.470904 | -4.955 | 0.377 | 4.993 | 8.214 | 2.424 | 4.84 | NaN | NaN | NaN | NaN | NaN | NaN | 25.948 | 18.467 | 14.374 | 12.932 | 16.441 | 14.66 | -5.102 | 1.186 | @:KKM3TI | ||
Consumer Discretionary | Consumer Discretionary | Consumer Discretionary | M1CD | 58.928 | 33.173 | 25.395 | 21.756 | 30.087 | 26.429 | -36.663 | 77.638 | 30.626 | 17.235 | -1.60729 | 0.427278 | 0.829197 | 1.26232 | 7.852 | 13.949 | 18.221 | 21.269 | 15.379 | 17.508 | NaN | NaN | NaN | NaN | NaN | NaN | 18.65 | 15.512 | 12.861 | 12.701 | 14.517 | 13.245 | 15.6538 | 1.296 | @:KKM1CD |
Automobiles & Components | Automobiles & Components | M2AC | 51.593 | 19.646 | 15.871 | 14.223 | 18.203 | 16.396 | -53.414 | 162.616 | 23.783 | 11.586 | -0.96591 | 0.120812 | 0.667325 | 1.2276 | 6.271 | 16.467 | 20.384 | 22.745 | 17.773 | 19.731 | NaN | NaN | NaN | NaN | NaN | NaN | 10.494 | 7.109 | 6 | 6.017 | 6.705 | 6.165 | 12.4352 | 1.098 | @:KKM2AC | |
Auto Components | M3AU | 58.453 | 16.917 | 12.85 | 11.104 | 15.303 | 13.387 | -72.618 | 245.535 | 31.644 | 15.725 | -0.80494 | 0.068899 | 0.40608 | 0.706137 | 5.102 | 17.629 | 23.208 | 26.857 | 19.489 | 22.278 | NaN | NaN | NaN | NaN | NaN | NaN | 9.347 | 7.252 | 6.229 | 5.701 | 6.879 | 6.38 | 9.0395 | 1.305 | @:KKM3AU | ||
Automobiles | M3AM | 50.573 | 20.206 | 16.539 | 14.945 | 18.816 | 17.055 | -47.99 | 150.287 | 22.169 | 10.671 | -1.05382 | 0.134449 | 0.746042 | 1.40052 | 6.077 | 15.21 | 18.582 | 20.564 | 16.334 | 18.02 | NaN | NaN | NaN | NaN | NaN | NaN | 10.734 | 7.074 | 5.945 | 6.091 | 6.663 | 6.113 | 15.311 | 1.039 | @:KKM3AM | ||
Consumer Durables & Apparel | Consumer Durables & Apparel | M2CA | 39.496 | 24.894 | 21.827 | 19.543 | 23.978 | 22.45 | -17.331 | 58.657 | 14.053 | 12.841 | -2.27892 | 0.424399 | 1.55319 | 1.52192 | 10.379 | 16.467 | 18.781 | 20.975 | 17.096 | 18.259 | NaN | NaN | NaN | NaN | NaN | NaN | 17.608 | 14.899 | 13.523 | 20.34 | 14.542 | 13.896 | 24.3377 | 1.215 | @:KKM2CA | |
Household Durables | M3HD | 16.499 | 11.858 | 11.062 | 9.949 | 11.498 | 11.118 | 5.999 | 39.14 | 7.193 | 9.284 | 2.75029 | 0.302964 | 1.53788 | 1.07163 | 12.093 | 16.826 | 18.036 | 20.055 | 17.353 | 17.945 | NaN | NaN | NaN | NaN | NaN | NaN | 9.087 | 7.281 | 7.207 | 6.349 | 7.314 | 7.361 | 37.6744 | 2.044 | @:KKM3HD | ||
Leisure Equipment & Products | M3LE | 92.408 | 57.402 | 45.055 | 35.573 | 49.294 | 43.267 | -8.333 | 60.984 | 27.405 | 26.655 | -11.0894 | 0.941263 | 1.64404 | 1.33457 | 0.699 | 1.126 | 1.434 | 1.816 | 1.311 | 1.493 | NaN | NaN | NaN | NaN | NaN | NaN | 27.108 | 36.642 | 31.144 | 25.152 | 33.198 | 29.71 | 9.3023 | 2.776 | @:KKM3LE | ||
Textiles & Apparel | M3TA | 60.762 | 34.127 | 28.674 | 24.988 | 32.813 | 30.05 | -32.413 | 78.045 | 19.019 | 14.749 | -1.87462 | 0.437273 | 1.50765 | 1.69422 | 18.035 | 32.111 | 38.218 | 43.854 | 33.396 | 36.467 | NaN | NaN | NaN | NaN | NaN | NaN | 22.419 | 18.948 | 16.513 | 26.625 | 18.335 | 17.11 | 17.9191 | 0.995 | @:KKM3TA | ||
Hotels, Restaurants & Leisure | Hotels, Restaurants & Leisure | M2HR | NaN | 83.77 | 30.087 | 23.275 | 50.01 | 32.791 | -116.019 | NaN | 178.421 | 29.266 | NaN | NaN | 0.168629 | 0.795291 | -2.802 | 4.683 | 13.038 | 16.854 | 7.844 | 11.963 | NaN | NaN | NaN | NaN | NaN | NaN | 41.618 | 25.515 | 16.38 | 14.328 | 20.997 | 17.149 | 11.0818 | 1.122 | @:KKM2HR | |
Hotels Restaurants & Leisure | M3HR | NaN | 83.77 | 30.087 | 23.275 | 50.01 | 32.791 | -116.019 | NaN | 178.421 | 29.266 | NaN | NaN | 0.168629 | 0.795291 | -3.069 | 5.13 | 14.282 | 18.462 | 8.592 | 13.104 | NaN | NaN | NaN | NaN | NaN | NaN | 41.619 | 25.515 | 16.38 | 14.328 | 20.997 | 17.148 | 11.0818 | 1.122 | @:KKM3HR | ||
Media | Media | M2MD | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM2MD | |
Media | M3ME | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM3ME | ||
Retailing | Retailing | M2RT | 46.823 | 39.315 | 32.45 | 27.415 | 36.962 | 33.604 | 21.338 | 19.096 | 21.156 | 19.192 | 2.19435 | 2.05881 | 1.53384 | 1.42846 | 20.305 | 24.182 | 29.298 | 34.679 | 25.722 | 28.293 | NaN | NaN | NaN | NaN | NaN | NaN | 20.891 | 19.816 | 16.779 | 15.114 | 18.749 | 17.254 | 15.0235 | 1.578 | @:KKM2RT | |
Distributors | M3DI | 25.655 | 22.032 | 20.206 | 19.126 | 21.388 | 20.489 | 5.732 | 16.447 | 9.035 | 5.645 | 4.47575 | 1.33958 | 2.23641 | 3.38813 | 5.995 | 6.982 | 7.612 | 8.042 | 7.192 | 7.507 | NaN | NaN | NaN | NaN | NaN | NaN | 14.482 | 13.767 | 12.629 | NaN | 13.371 | 12.809 | 79.3103 | 1.626 | @:KKM3DI | ||
Internet & Catalog Retail | M3NT | 82.281 | 70.084 | 50.214 | 37.414 | 62.269 | 52.959 | 91.499 | 17.404 | 39.57 | 34.214 | 0.899256 | 4.02689 | 1.26899 | 1.09353 | 53.791 | 63.152 | 88.142 | 118.298 | 71.078 | 83.573 | NaN | NaN | NaN | NaN | NaN | NaN | 30.253 | 27.479 | 20.878 | 17.578 | 24.935 | 21.79 | -1.5748 | 1.155 | @:KKM3NT | ||
Multiline Retail | M3MR | 22.783 | 22.275 | 20.3 | 19.213 | 21.752 | 20.718 | 25.087 | 2.28 | 9.731 | 7.532 | 0.90816 | 9.76974 | 2.08612 | 2.55085 | 17.945 | 18.354 | 20.14 | 21.279 | 18.796 | 19.733 | NaN | NaN | NaN | NaN | NaN | NaN | 11.417 | 11.744 | 11.212 | 10.993 | 11.601 | 11.305 | 7.6923 | 1.453 | @:KKM3MR | ||
Specialty Retail | M3SR | 31.45 | 24.688 | 21.866 | 19.79 | 23.831 | 22.428 | -4.976 | 27.39 | 12.908 | 10.487 | -6.32034 | 0.901351 | 1.69399 | 1.8871 | 14.657 | 18.672 | 21.082 | 23.293 | 19.344 | 20.554 | NaN | NaN | NaN | NaN | NaN | NaN | 15.747 | 14.874 | 13.839 | 12.946 | 14.569 | 14.042 | 23.0047 | 1.64 | @:KKM3SR | ||
Consumer Staples | Consumer Staples | Consumer Staples | M1CS | 22.224 | 20.903 | 19.214 | 17.937 | 20.148 | 19.343 | -2.068 | 6.321 | 8.789 | 7.646 | -10.7466 | 3.30691 | 2.18614 | 2.34593 | 12.453 | 13.24 | 14.404 | 15.429 | 13.736 | 14.308 | NaN | NaN | NaN | NaN | NaN | NaN | 12.989 | 13.258 | 12.341 | 11.988 | 12.875 | 12.461 | 5.7784 | 2.653 | @:KKM1CS |
Food & Staples Retailing | Food & Staples Retailing | M2FD | 21.674 | 21.304 | 19.216 | 18.101 | 20.18 | 19.315 | 1.165 | 1.739 | 10.865 | 8.023 | 18.6043 | 12.2507 | 1.76861 | 2.25614 | 7.408 | 7.537 | 8.355 | 8.87 | 7.956 | 8.313 | NaN | NaN | NaN | NaN | NaN | NaN | 9.623 | 9.844 | 8.854 | 9.196 | 9.412 | 9.052 | 2.0772 | 1.943 | @:KKM2FD | |
Food & Staples Retailing | M3FD | 21.674 | 21.304 | 19.216 | 18.101 | 20.18 | 19.315 | 1.165 | 1.739 | 10.865 | 8.023 | 18.6043 | 12.2507 | 1.76861 | 2.25614 | 7.408 | 7.537 | 8.355 | 8.87 | 7.956 | 8.313 | NaN | NaN | NaN | NaN | NaN | NaN | 9.623 | 9.844 | 8.854 | 9.196 | 9.412 | 9.052 | 2.0772 | 1.943 | @:KKM3FD | ||
Food Beverage & Tobacco | Food Beverage & Tobacco | M2FB | 21.252 | 19.677 | 18.085 | 16.805 | 19.059 | 18.283 | -4.647 | 8 | 8.807 | 7.824 | -4.57327 | 2.45962 | 2.05348 | 2.14788 | 14.98 | 16.178 | 17.603 | 18.943 | 16.703 | 17.412 | NaN | NaN | NaN | NaN | NaN | NaN | 13.968 | 13.556 | 12.651 | 12.024 | 13.214 | 12.775 | 11.2885 | 2.988 | @:KKM2FB | |
Beverages | M3BV | 28.699 | 25.411 | 22.808 | 20.836 | 24.288 | 23.05 | -12.886 | 12.94 | 11.409 | 9.469 | -2.22715 | 1.96376 | 1.99912 | 2.20044 | 10.879 | 12.287 | 13.689 | 14.985 | 12.855 | 13.546 | NaN | NaN | NaN | NaN | NaN | NaN | 17.363 | 16.471 | 14.985 | 14.325 | 15.858 | 15.148 | 13.8667 | 2.268 | @:KKM3BV | ||
Food Products | M3FP | 23.178 | 21.801 | 20.185 | 19.155 | 21.209 | 20.418 | -2.089 | 6.313 | 8.008 | 6.433 | -11.0953 | 3.45335 | 2.5206 | 2.97762 | 13.16 | 13.991 | 15.111 | 15.924 | 14.382 | 14.938 | NaN | NaN | NaN | NaN | NaN | NaN | 14.535 | 13.866 | 13.12 | 12.759 | 13.607 | 13.246 | 7.7295 | 2.297 | @:KKM3FP | ||
Tobacco | M3TB | 11.53 | 10.933 | 10.2 | 9.459 | 10.673 | 10.307 | 1.092 | 5.464 | 7.183 | 7.831 | 10.5586 | 2.00092 | 1.42002 | 1.20789 | 31.013 | 32.707 | 35.056 | 37.801 | 33.501 | 34.693 | NaN | NaN | NaN | NaN | NaN | NaN | 9.075 | 9.434 | 8.923 | 8.336 | 9.254 | 8.998 | 17.0455 | 6.461 | @:KKM3TB | ||
Household & Personal Products | Household & Personal Products | M2HH | 25.369 | 24.013 | 22.438 | 21.001 | 23.167 | 22.35 | 2.737 | 5.647 | 7.019 | 6.841 | 9.26891 | 4.25235 | 3.19675 | 3.06987 | 12.939 | 13.67 | 14.63 | 15.63 | 14.169 | 14.687 | NaN | NaN | NaN | NaN | NaN | NaN | 14.612 | 16.74 | 15.872 | 15.13 | 16.299 | 15.865 | -8.1712 | 2.421 | @:KKM2HH | |
Household Products | M3HP | 23.577 | 22.34 | 20.964 | 19.697 | 21.553 | 20.821 | 7.146 | 5.54 | 6.563 | 6.434 | 3.29933 | 4.03249 | 3.19427 | 3.06139 | 12.993 | 13.712 | 14.612 | 15.552 | 14.213 | 14.713 | NaN | NaN | NaN | NaN | NaN | NaN | 15.414 | 15.735 | 15.096 | 14.314 | 15.428 | 15.034 | -23.5669 | 2.566 | @:KKM3HP | ||
Personal Products | M3PP | 29.354 | 27.722 | 25.661 | 23.82 | 26.746 | 25.726 | -5.876 | 5.887 | 8.03 | 7.73 | -4.99558 | 4.70902 | 3.19564 | 3.0815 | 13.384 | 14.172 | 15.31 | 16.494 | 14.689 | 15.272 | NaN | NaN | NaN | NaN | NaN | NaN | 12.985 | 18.664 | 17.33 | 16.657 | 17.951 | 17.432 | 16 | 2.162 | @:KKM3PP | ||
Health Care | Health Care | Health Care | M1HC | 20.773 | 18.076 | 16.795 | 15.617 | 17.652 | 17.003 | 5.684 | 14.924 | 7.628 | 7.26 | 3.65464 | 1.2112 | 2.20176 | 2.1511 | 15.82 | 18.181 | 19.568 | 21.044 | 18.618 | 19.328 | NaN | NaN | NaN | NaN | NaN | NaN | 14.201 | 13.127 | 12.059 | 11.091 | 12.766 | 12.225 | 9.2046 | 2.162 | @:KKM1HC |
Health Care Equipment & Services | Health Care Equipment & Services | M2HE | 26.702 | 22.633 | 20.717 | 18.617 | 22.057 | 21.075 | 5.235 | 17.977 | 9.246 | 10.781 | 5.10067 | 1.259 | 2.24064 | 1.72683 | 28.196 | 33.264 | 36.34 | 40.44 | 34.132 | 35.723 | NaN | NaN | NaN | NaN | NaN | NaN | 15.404 | 14.631 | 13.318 | 12.162 | 14.209 | 13.532 | 16.3949 | 1.215 | @:KKM2HE | |
Health Care Equipment & Supplies | M3HS | 40.8 | 30.91 | 28.421 | 25.64 | 30.267 | 28.973 | -2.203 | 31.996 | 8.759 | 10.518 | -18.5202 | 0.966058 | 3.24478 | 2.43773 | 17.679 | 23.335 | 25.379 | 28.132 | 23.831 | 24.896 | NaN | NaN | NaN | NaN | NaN | NaN | 24.441 | 20.84 | 18.854 | 17.352 | 20.268 | 19.238 | 19.0259 | 1.034 | @:KKM3HS | ||
Health Care Providers & Services | M3PS | 16.564 | 15.279 | 13.991 | 12.615 | 14.856 | 14.2 | 11.331 | 8.411 | 9.207 | 10.765 | 1.46183 | 1.81655 | 1.5196 | 1.17185 | 48.581 | 52.667 | 57.516 | 63.787 | 54.168 | 56.668 | NaN | NaN | NaN | NaN | NaN | NaN | 9.902 | 10.143 | 9.292 | 8.386 | 9.845 | 9.405 | 18.7831 | 1.446 | @:KKM3PS | ||
Pharmaceuticals, Biotechnology & Life Sciences | Pharmaceuticals, Biotechnology & Life Sciences | M2PB | 17.9 | 15.778 | 14.772 | 14.007 | 15.425 | 14.919 | 5.903 | 13.444 | 6.813 | 5.461 | 3.03236 | 1.17361 | 2.16821 | 2.56491 | 13.408 | 15.211 | 16.247 | 17.135 | 15.56 | 16.087 | NaN | NaN | NaN | NaN | NaN | NaN | 13.471 | 12.253 | 11.316 | 10.445 | 11.925 | 11.457 | 2.2046 | 2.814 | @:KKM2PB | |
Biotechnology | M3BI | 17.357 | 14.882 | 14.049 | 14.11 | 14.588 | 14.147 | 14.739 | 16.634 | 5.925 | -0.431 | 1.17762 | 0.894674 | 2.37114 | -32.7378 | 79.428 | 92.64 | 98.129 | 97.706 | 94.508 | 97.455 | NaN | NaN | NaN | NaN | NaN | NaN | 13.374 | 11.588 | 10.52 | 9.693 | 11.22 | 10.669 | -8.0729 | 3.459 | @:KKM3BI | ||
Pharmaceuticals | M3PH | 16.3 | 14.573 | 13.492 | 12.6 | 14.193 | 13.659 | 1.148 | 11.85 | 8.017 | 7.072 | 14.1986 | 1.22979 | 1.68292 | 1.78167 | 10.453 | 11.692 | 12.629 | 13.522 | 12.005 | 12.474 | NaN | NaN | NaN | NaN | NaN | NaN | 12.577 | 11.538 | 10.589 | 9.911 | 11.204 | 10.736 | -2.5591 | 3.029 | @:KKM3PH | ||
Financials | Financials | Financials | M1FN | 18.188 | 13.451 | 12.756 | 11.593 | 13.194 | 12.853 | -21.036 | 35.304 | 5.408 | 10.197 | -0.86461 | 0.381005 | 2.35873 | 1.1369 | 8.114 | 10.972 | 11.57 | 12.73 | 11.186 | 11.483 | NaN | NaN | NaN | NaN | NaN | NaN | 10.416 | 11.49 | 10.478 | 10.368 | 11.883 | 11.666 | 22.0286 | 2.308 | @:KKM1FN |
Banks | Banks | M2B2 | 17.68 | 11.913 | 11.521 | 10.397 | 11.756 | 11.561 | -34.349 | 48.413 | 3.399 | 10.811 | -0.51472 | 0.24607 | 3.38953 | 0.961706 | 6.262 | 9.293 | 9.609 | 10.648 | 9.417 | 9.576 | NaN | NaN | NaN | NaN | NaN | NaN | 6.124 | 17.066 | 5.678 | 5.159 | 16.844 | 16.52 | 30.6991 | 2.347 | @:KKM2B2 | |
M3B3 | 17.68 | 11.913 | 11.521 | 10.397 | 11.756 | 11.561 | -34.349 | 48.413 | 3.399 | 10.811 | -0.51472 | 0.24607 | 3.38953 | 0.961706 | 7.029 | 10.432 | 10.787 | 11.953 | 10.572 | 10.75 | NaN | NaN | NaN | NaN | NaN | NaN | 6.124 | 17.066 | 5.678 | 5.159 | 16.843 | 16.518 | 30.6991 | 2.347 | @:KKM3B3 | |||
Diversified Financials | Diversified Financials | M2D2 | 21.441 | 16.666 | 15.937 | 14.515 | 16.421 | 16.058 | 0.779 | 28.861 | 4.544 | 9.921 | 27.5237 | 0.577457 | 3.50726 | 1.46306 | 9.937 | 12.785 | 13.369 | 14.679 | 12.975 | 13.269 | NaN | NaN | NaN | NaN | NaN | NaN | 13.125 | 11.68 | 12.407 | 12.471 | 12.45 | 12.366 | 27.7835 | 1.743 | @:KKM2D2 | |
Diversified Financial Services | M3D3 | 22.08 | 22.003 | 20.207 | 19.422 | 21.441 | 20.502 | -5.555 | 2.518 | 9.449 | 5.221 | -3.9748 | 8.73828 | 2.13853 | 3.71998 | 8.472 | 8.501 | 9.257 | 9.632 | 8.725 | 9.124 | NaN | NaN | NaN | NaN | NaN | NaN | 4.171 | 12.159 | 18.297 | 22.226 | 13.454 | 16.02 | 2.7778 | 2.205 | @:KKM3D3 | ||
Insurance | Insurance | M2I2 | 15.604 | 13.018 | 11.77 | 10.817 | 12.563 | 11.956 | -10.837 | 19.865 | 10.603 | 9.244 | -1.43988 | 0.655323 | 1.11006 | 1.17016 | 8.936 | 10.712 | 11.847 | 12.891 | 11.1 | 11.664 | NaN | NaN | NaN | NaN | NaN | NaN | 6.659 | 10.74 | 8.204 | 8.027 | 10.054 | 9.496 | 6.3123 | 2.946 | @:KKM2I2 | |
Insurance | M3I3 | 15.604 | 13.018 | 11.77 | 10.817 | 12.563 | 11.956 | -10.837 | 19.865 | 10.603 | 9.244 | -1.43988 | 0.655323 | 1.11006 | 1.17016 | 8.936 | 10.712 | 11.847 | 12.891 | 11.1 | 11.664 | NaN | NaN | NaN | NaN | NaN | NaN | 6.659 | 10.74 | 8.204 | 8.027 | 10.054 | 9.496 | 6.3123 | 2.946 | @:KKM3I3 | ||
Real Estate | Real Estate | Real Estate | M1RE | 36.133 | 33.914 | 30.25 | 27.612 | 32.437 | 30.644 | -21.715 | 6.544 | 12.112 | 10.911 | -1.66397 | 5.18246 | 2.49752 | 2.53066 | 32.691 | 34.831 | 39.049 | 42.78 | 36.417 | 38.547 | NaN | NaN | NaN | NaN | NaN | NaN | 20.855 | 20.721 | 19.281 | 18.252 | 20.155 | 19.459 | 5.9977 | 2.843 | @:KKM1RE |
Information Technology | Information Technology | Information Technology | M1IT | 37.748 | 30.039 | 26.845 | 24.37 | 28.335 | 26.841 | 6.814 | 25.662 | 11.9 | 10.227 | 5.53977 | 1.17056 | 2.25588 | 2.38291 | 13.597 | 17.086 | 19.12 | 21.061 | 18.114 | 19.122 | NaN | NaN | NaN | NaN | NaN | NaN | 20.718 | 19.898 | 18.211 | 16.735 | 18.997 | 18.15 | 15.8019 | 1.057 | @:KKM1IT |
Software & Services | Software & Services | M2SS | 45.52 | 38.507 | 33.759 | 29.245 | 36.136 | 33.62 | 6.832 | 18.212 | 14.065 | 15.399 | 6.66276 | 2.11438 | 2.40021 | 1.89915 | 12.943 | 15.3 | 17.452 | 20.145 | 16.304 | 17.524 | NaN | NaN | NaN | NaN | NaN | NaN | 25.324 | 25.142 | 22.474 | 20.2 | 23.642 | 22.238 | 7.9109 | 1.047 | @:KKM2SS | |
Internet Software & Services | M3NS | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM3NS | ||
IT Consulting & Services | M3IS | 45.992 | 38.853 | 32.506 | 27.986 | 35.945 | 32.975 | -10.357 | 18.375 | 19.524 | 16.287 | -4.44067 | 2.11445 | 1.66493 | 1.7183 | 5.591 | 6.619 | 7.911 | 9.188 | 7.154 | 7.798 | NaN | NaN | NaN | NaN | NaN | NaN | 24.436 | 24.713 | 21.993 | 19.288 | 23.406 | 22.188 | 5.2567 | 1.195 | @:KKM3IS | ||
Software | M3SW | 45.212 | 38.281 | 34.643 | 30.135 | 36.264 | 34.063 | 22.094 | 18.105 | 10.501 | 14.779 | 2.04635 | 2.11439 | 3.29902 | 2.03904 | 15.781 | 18.639 | 20.596 | 23.677 | 19.675 | 20.947 | NaN | NaN | NaN | NaN | NaN | NaN | 26.156 | 25.777 | 23.112 | 21.106 | 24.093 | 22.568 | 10.1331 | 0.956 | @:KKM3SW | ||
Technology Hardware & Equipment | Technology Hardware & Equipment | M2TH | 32.33 | 24.02 | 22.481 | 21.405 | 23.122 | 22.447 | 6.127 | 34.594 | 6.846 | 5.092 | 5.27664 | 0.69434 | 3.28382 | 4.20365 | 15.139 | 20.377 | 21.772 | 22.866 | 21.169 | 21.805 | NaN | NaN | NaN | NaN | NaN | NaN | 17.952 | 15.71 | 15.234 | 14.301 | 15.477 | 15.217 | 25.4369 | 0.916 | @:KKM2TH | |
Communications Equipment | M3CE | 17.311 | 17.305 | 15.71 | 14.667 | 16.446 | 15.718 | 5.552 | 0.039 | 10.154 | 7.105 | 3.11798 | 443.718 | 1.54717 | 2.06432 | 5.03 | 5.032 | 5.543 | 5.937 | 5.295 | 5.54 | NaN | NaN | NaN | NaN | NaN | NaN | 9.622 | 10.848 | 9.912 | 9.363 | 10.398 | 9.973 | 14.8649 | 2.394 | @:KKM3CE | ||
Computers & Peripherals | M3CP | 36.664 | 25.09 | 23.742 | 22.894 | 24.225 | 23.66 | 8.251 | 46.13 | 5.676 | 4.095 | 4.44358 | 0.543898 | 4.18288 | 5.59072 | 39.11 | 57.151 | 60.395 | 62.632 | 59.191 | 60.606 | NaN | NaN | NaN | NaN | NaN | NaN | 20.696 | 16.842 | 16.623 | 15.683 | 16.705 | 16.562 | 30.5263 | 0.695 | @:KKM3CP | ||
Electrical Equipment | M3EE | 39.488 | 29.788 | 25.659 | 22.997 | 27.976 | 26.015 | -21.317 | 32.564 | 16.091 | 11.573 | -1.85242 | 0.914752 | 1.59462 | 1.98713 | 12.121 | 16.069 | 18.654 | 20.813 | 17.109 | 18.399 | NaN | NaN | NaN | NaN | NaN | NaN | 18.721 | 17.625 | 15.216 | 14.024 | 16.62 | 15.433 | 21.0191 | 1.802 | @:KKM3EE | ||
Semiconductors & Semiconductor Equipment | M3SC | 30.655 | 23.983 | 20.864 | 19.278 | 22.228 | 21.033 | 7.648 | 27.819 | 14.952 | 8.012 | 4.00824 | 0.862109 | 1.3954 | 2.40614 | 24.361 | 31.138 | 35.794 | 38.738 | 33.597 | 35.506 | NaN | NaN | NaN | NaN | NaN | NaN | 15.988 | 16.402 | 14.498 | 13.561 | 15.426 | 14.597 | 29.7872 | 1.276 | @:KKM3SC | ||
Telecommunication Services | Telecommunication Services | Telecommunication Services | M1T1 | 28.425 | 23.756 | 20.627 | 17.992 | 22.492 | 21.005 | -1.625 | 19.657 | 15.171 | 14.691 | -17.4923 | 1.20853 | 1.35963 | 1.2247 | 3.402 | 4.071 | 4.688 | 5.375 | 4.299 | 4.604 | NaN | NaN | NaN | NaN | NaN | NaN | 11.184 | 11.288 | 9.766 | 9.564 | 10.704 | 9.97 | 10.5736 | 3.021 | @:KKM1T1 |
M2T2 | 13.524 | 13.04 | 12.425 | 11.494 | 12.861 | 12.552 | -5.337 | 3.716 | 4.944 | 7.867 | -2.53401 | 3.50915 | 2.51315 | 1.46104 | 4.423 | 4.587 | 4.814 | 5.205 | 4.651 | 4.766 | NaN | NaN | NaN | NaN | NaN | NaN | 6.605 | 6.709 | 6.655 | 6.245 | 6.7 | 6.673 | 0.7092 | 5.202 | @:KKM2T2 | |||
Diversified Telecommunications Services | M3DT | 12.326 | 11.976 | 11.565 | 10.959 | 11.844 | 11.636 | -5.364 | 2.917 | 3.558 | 5.12 | -2.29791 | 4.10559 | 3.25042 | 2.14043 | 4.117 | 4.237 | 4.388 | 4.63 | 4.284 | 4.361 | NaN | NaN | NaN | NaN | NaN | NaN | 6.442 | 6.606 | 6.565 | 6.184 | 6.595 | 6.574 | -0.6148 | 5.195 | @:KKM3DT | ||
Wireless Telecommunication Services | M3WT | 27.564 | 24.378 | 20.363 | 15.335 | 23.369 | 21.351 | -5.017 | 13.069 | 19.721 | 32.786 | -5.49412 | 1.86533 | 1.03255 | 0.46773 | 3.953 | 4.47 | 5.351 | 7.105 | 4.663 | 5.103 | NaN | NaN | NaN | NaN | NaN | NaN | 7.615 | 7.321 | 7.186 | 6.588 | 7.327 | 7.259 | 9.2105 | 5.277 | @:KKM3WT | ||
Utilities | Utilities | Utilities | M1U1 | 19.71 | 18.857 | 17.477 | 16.657 | 18.396 | 17.707 | -2.387 | 4.523 | 7.892 | 4.925 | -8.25723 | 4.16914 | 2.21452 | 3.38213 | 8.694 | 9.087 | 9.804 | 10.287 | 9.315 | 9.677 | NaN | NaN | NaN | NaN | NaN | NaN | 11.258 | 10.9 | 10.544 | 10.382 | 10.765 | 10.587 | 1.2915 | 3.526 | @:KKM1U1 |
M2U2 | 19.71 | 18.857 | 17.477 | 16.657 | 18.396 | 17.707 | -2.387 | 4.523 | 7.892 | 4.925 | -8.25723 | 4.16914 | 2.21452 | 3.38213 | 8.694 | 9.087 | 9.804 | 10.287 | 9.315 | 9.677 | NaN | NaN | NaN | NaN | NaN | NaN | 11.258 | 10.9 | 10.544 | 10.382 | 10.765 | 10.587 | 1.2915 | 3.526 | @:KKM2U2 | |||
Electric Utilities | M3EU | 19.302 | 18.744 | 17.591 | 16.734 | 18.346 | 17.776 | -2.881 | 2.978 | 6.556 | 5.117 | -6.69976 | 6.29416 | 2.68319 | 3.27028 | 11.598 | 11.943 | 12.726 | 13.377 | 12.202 | 12.593 | NaN | NaN | NaN | NaN | NaN | NaN | 11.176 | 11.036 | 10.71 | 10.517 | 10.924 | 10.763 | -2.1544 | 3.527 | @:KKM3EU | ||
Gas Utilities | M3GU | 20.194 | 18.64 | 17.729 | 17.078 | 18.12 | 17.708 | -1.761 | 8.335 | 5.142 | 3.808 | -11.4673 | 2.23635 | 3.44788 | 4.48477 | 14.695 | 15.92 | 16.739 | 17.376 | 16.377 | 16.758 | NaN | NaN | NaN | NaN | NaN | NaN | 11.167 | 11.171 | 10.845 | 10.579 | 10.987 | 10.84 | 6.7308 | 4.292 | @:KKM3GU | ||
Multi-Utilities | M3MU | 19.812 | 17.869 | 16.638 | 15.828 | 17.518 | 16.878 | -0.234 | 10.874 | 7.398 | 5.115 | -84.6667 | 1.64328 | 2.24899 | 3.09443 | 3.01 | 3.337 | 3.584 | 3.767 | 3.404 | 3.533 | NaN | NaN | NaN | NaN | NaN | NaN | 11.306 | 10.295 | 10.087 | 9.898 | 10.186 | 10.077 | 5.414 | 3.583 | @:KKM3MU | ||
Water Utilities | M3WU | 29.442 | 27.446 | 24.938 | 24.373 | 26.912 | 25.648 | -8.524 | 7.273 | 10.057 | 2.319 | -3.45401 | 3.77368 | 2.47967 | 10.5101 | 16.443 | 17.639 | 19.413 | 19.863 | 17.989 | 18.876 | NaN | NaN | NaN | NaN | NaN | NaN | 17.429 | 15.54 | 14.781 | 14.904 | 15.375 | 14.994 | 8.3333 | 2.455 | @:KKM3WU |
Now let's see our data-frame in the list:
|
PE-Ratio | Earnings Growth | PEG-Ratio | EPS Mean | Risk premium | EV/EBITDA | Rev. Ratio | Div Yield | Tickers | |||||||||||||||||||||||||||||
Fiscal Year | Forward | Fiscal Year | Fiscal Year | Fiscal Year | Forward | Fiscal Year | Forward | Fiscal Year | Forward | Rev. Ratio | Div Yield | Tickers | ||||||||||||||||||||||||||
FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | FY0 | FY1 | FY2 | FY3 | FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | 12M | 18M | FY0 | FY1 | FY2 | FY3 | 12M | 18M | Rev. Ratio | Div Yield | Tickers | ||||
Energy | Energy | Energy | M1E1 | 460.023 | 16.258 | 13.12 | 12.335 | 15.046 | 13.549 | -96.896 | 2729.53 | 23.914 | 6.369 | -4.7476 | 0.005956 | 0.548633 | 1.93672 | 0.336 | 9.515 | 11.791 | 12.542 | 10.282 | 11.418 | NaN | NaN | NaN | NaN | NaN | NaN | 9.515 | 6.273 | 5.54 | 5.25 | 6.011 | 5.652 | 15.0922 | 4.567 | @:KKM1E1 |
M2E2 | 460.023 | 16.258 | 13.12 | 12.335 | 15.046 | 13.549 | -96.896 | 2729.53 | 23.914 | 6.369 | -4.7476 | 0.005956 | 0.548633 | 1.93672 | 0.336 | 9.515 | 11.791 | 12.542 | 10.282 | 11.418 | NaN | NaN | NaN | NaN | NaN | NaN | 9.515 | 6.273 | 5.54 | 5.25 | 6.011 | 5.652 | 15.0922 | 4.567 | @:KKM2E2 | |||
Energy Equipment & Services | M3ES | 48.738 | 24.336 | 16.978 | 12.999 | 21.264 | 17.879 | -61.793 | 100.27 | 43.339 | 30.608 | -0.78873 | 0.242705 | 0.391749 | 0.424693 | 1.914 | 3.834 | 5.495 | 7.177 | 4.387 | 5.218 | NaN | NaN | NaN | NaN | NaN | NaN | 10.221 | 9.821 | 8.184 | 6.571 | 9.214 | 8.422 | 60 | 1.933 | @:KKM3ES | ||
Oil, Gas & Consumable Fuels | M3OG | 754.708 | 16.012 | 12.984 | 12.306 | 14.845 | 13.399 | -98.128 | 4613.39 | 23.323 | 5.512 | -7.69106 | 0.003471 | 0.556704 | 2.23258 | 0.216 | 10.202 | 12.582 | 13.275 | 11.004 | 12.192 | NaN | NaN | NaN | NaN | NaN | NaN | 9.485 | 6.171 | 5.458 | 5.204 | 5.917 | 5.567 | 10.2171 | 4.692 | @:KKM3OG | ||
Materials | Materials | Materials | M1M1 | 24.337 | 15.256 | 15.8 | 16.168 | 15.466 | 15.853 | -0.406 | 59.526 | -3.441 | -2.37 | -59.9433 | 0.256291 | -4.59169 | -6.82194 | 16.089 | 25.665 | 24.782 | 24.218 | 25.316 | 24.699 | NaN | NaN | NaN | NaN | NaN | NaN | 9.202 | 7.408 | 7.765 | 7.937 | 7.556 | 7.769 | 18.7947 | 2.466 | @:KKM1M1 |
M2M2 | 24.337 | 15.256 | 15.8 | 16.168 | 15.466 | 15.853 | -0.406 | 59.526 | -3.441 | -2.37 | -59.9433 | 0.256291 | -4.59169 | -6.82194 | 16.089 | 25.665 | 24.782 | 24.218 | 25.316 | 24.699 | NaN | NaN | NaN | NaN | NaN | NaN | 9.202 | 7.408 | 7.765 | 7.937 | 7.556 | 7.769 | 18.7947 | 2.466 | @:KKM2M2 | |||
Chemicals | M3CH | 30.134 | 22.245 | 20.789 | 19.053 | 21.712 | 21.001 | -11.668 | 35.463 | 7.007 | 9.111 | -2.58262 | 0.627273 | 2.96689 | 2.09121 | 16.695 | 22.616 | 24.2 | 26.405 | 23.171 | 23.956 | NaN | NaN | NaN | NaN | NaN | NaN | 13.859 | 12.249 | 11.695 | 11.045 | 12.05 | 11.776 | 23.3813 | 1.995 | @:KKM3CH | ||
Construction Materials | M3CM | 20.782 | 17.937 | 16.01 | 14.567 | 17.245 | 16.302 | -3.319 | 15.858 | 12.038 | 9.904 | -6.26152 | 1.1311 | 1.32996 | 1.47082 | 13.536 | 15.683 | 17.571 | 19.311 | 16.312 | 17.256 | NaN | NaN | NaN | NaN | NaN | NaN | 8.454 | 9.033 | 8.225 | 7.689 | 8.752 | 8.353 | 26.6055 | 2.156 | @:KKM3CM | ||
Containers & Packaging | M3CT | 21.836 | 18.241 | 16.117 | 14.806 | 17.247 | 16.267 | -9.876 | 19.711 | 13.18 | 7.342 | -2.21102 | 0.925422 | 1.22284 | 2.01662 | 16.238 | 19.439 | 22.001 | 23.949 | 20.56 | 21.798 | NaN | NaN | NaN | NaN | NaN | NaN | 10.344 | 10.207 | 9.158 | 8.866 | 9.755 | 9.254 | 16.6667 | 2 | @:KKM3CT | ||
Metals & Mining | M3MM | 20.305 | 10.422 | 11.899 | 13.816 | 10.942 | 11.869 | 15.7 | 94.834 | -12.412 | -13.877 | 1.29331 | 0.109897 | -0.95867 | -0.9956 | 20.513 | 39.966 | 35.006 | 30.148 | 38.068 | 35.092 | NaN | NaN | NaN | NaN | NaN | NaN | 6.593 | 4.943 | 5.55 | 6.002 | 5.173 | 5.521 | 7.6225 | 3.217 | @:KKM3MM | ||
Paper & Forest Products | M3PF | 21.168 | 14.034 | 16.437 | 16.372 | 14.753 | 15.981 | -4.005 | 50.832 | -14.619 | 0.397 | -5.28539 | 0.276086 | -1.12436 | 41.2393 | 9.832 | 14.829 | 12.661 | 12.711 | 14.106 | 13.023 | NaN | NaN | NaN | NaN | NaN | NaN | 10.491 | 8.528 | 9.35 | 9.269 | 8.784 | 9.201 | 50 | 2.498 | @:KKM3PF | ||
Industrials | Industrials | Industrials | M1ID | 38.012 | 25.669 | 21.344 | 18.795 | 23.949 | 21.891 | -32.919 | 48.085 | 20.323 | 13.077 | -1.15471 | 0.533826 | 1.05024 | 1.43726 | 9.494 | 14.059 | 16.908 | 19.201 | 15.069 | 16.485 | NaN | NaN | NaN | NaN | NaN | NaN | 15.635 | 14.22 | 12.389 | 11.402 | 13.514 | 12.63 | 23.5447 | 1.629 | @:KKM1ID |
Capital Goods | Capital Goods | M2CG | 35.588 | 24.677 | 20.62 | 18.26 | 23.051 | 21.114 | -35.761 | 44.217 | 19.677 | 13.021 | -0.99516 | 0.558089 | 1.04792 | 1.40235 | 9.65 | 13.917 | 16.656 | 18.808 | 14.899 | 16.266 | NaN | NaN | NaN | NaN | NaN | NaN | 14.874 | 14.13 | 12.239 | 11.201 | 13.395 | 12.476 | 22.0871 | 1.699 | @:KKM2CG | |
Aerospace & Defense | M3AD | 60.095 | 25.637 | 19.173 | 16.342 | 23.01 | 19.987 | -67.659 | 134.406 | 33.715 | 17.321 | -0.8882 | 0.190743 | 0.568679 | 0.943479 | 8.239 | 19.312 | 25.823 | 30.295 | 21.517 | 24.771 | NaN | NaN | NaN | NaN | NaN | NaN | 22.278 | 15.34 | 12.396 | 10.799 | 14.215 | 12.799 | 6.5527 | 1.45 | @:KKM3AD | ||
Building Products | M3BP | 27.988 | 23.267 | 20.783 | 18.933 | 22.249 | 21.052 | -4.169 | 20.292 | 11.95 | 9.772 | -6.71336 | 1.14661 | 1.73916 | 1.93747 | 12.157 | 14.624 | 16.371 | 17.971 | 15.292 | 16.162 | NaN | NaN | NaN | NaN | NaN | NaN | 12.955 | 13.177 | 12.122 | 11.324 | 12.766 | 12.254 | 33.7121 | 1.412 | @:KKM3BP | ||
Construction & Engineering | M3CN | 29.554 | 20.199 | 15.902 | 14.193 | 18.53 | 16.486 | -44.204 | 46.317 | 27.02 | 12.038 | -0.66858 | 0.436103 | 0.588527 | 1.17902 | 16.039 | 23.468 | 29.809 | 33.398 | 25.582 | 28.753 | NaN | NaN | NaN | NaN | NaN | NaN | 8.124 | 8.025 | 6.953 | 7.127 | 7.641 | 7.116 | 1.7544 | 2.972 | @:KKM3CN | ||
Electronic Equipment & Instruments | M3EI | 34.149 | 26.911 | 24.233 | 21.679 | 25.82 | 24.509 | -6.623 | 26.896 | 11.049 | 9.805 | -5.15612 | 1.00056 | 2.19323 | 2.21101 | 3.505 | 4.448 | 4.94 | 5.521 | 4.636 | 4.884 | NaN | NaN | NaN | NaN | NaN | NaN | 15.629 | 15.039 | 13.917 | 12.775 | 14.612 | 14.035 | 28.8136 | 1.196 | @:KKM3EI | ||
Industrial Conglomerates | M3IC | 30.95 | 22.84 | 19.637 | 17.362 | 21.517 | 19.979 | -33.445 | 35.507 | 16.311 | 13.54 | -0.9254 | 0.643253 | 1.20391 | 1.28227 | 4.73 | 6.409 | 7.455 | 8.432 | 6.804 | 7.328 | NaN | NaN | NaN | NaN | NaN | NaN | 11.825 | 14.08 | 12.15 | 10.731 | 13.265 | 12.345 | 25.7485 | 1.836 | @:KKM3IC | ||
Machinery | M3MC | 32.562 | 24.616 | 21.305 | 19.227 | 23.285 | 21.699 | -22.564 | 32.28 | 15.542 | 10.806 | -1.4431 | 0.762577 | 1.3708 | 1.77929 | 21.943 | 29.026 | 33.537 | 37.161 | 30.685 | 32.928 | NaN | NaN | NaN | NaN | NaN | NaN | 15.024 | 14.263 | 12.787 | 12.251 | 13.689 | 12.969 | 31.4332 | 1.683 | @:KKM3MC | ||
Trading Companies & Distributors Industry | M3TC | 25.016 | 22.991 | 20.446 | 18.214 | 22.165 | 20.911 | -1.684 | 8.81 | 12.449 | 12.25 | -14.8551 | 2.60965 | 1.64238 | 1.48686 | 20.242 | 22.025 | 24.767 | 27.801 | 22.846 | 24.216 | NaN | NaN | NaN | NaN | NaN | NaN | 10.657 | 11.443 | 10.381 | 9.289 | 11.086 | 10.558 | 13.3333 | 1.738 | @:KKM3TC | ||
Commercial Services & Supplies | Commercial Services & Supplies | M2C2 | 33.587 | 29.412 | 26.122 | 22.809 | 28.183 | 26.573 | -3.289 | 14.194 | 12.597 | 12.324 | -10.2119 | 2.07214 | 2.07367 | 1.85078 | 8.029 | 9.169 | 10.324 | 11.823 | 9.569 | 10.149 | NaN | NaN | NaN | NaN | NaN | NaN | 17.198 | 16.23 | 14.534 | 13.315 | 15.649 | 14.811 | 25.1641 | 1.464 | @:KKM2C2 | |
Commercial Services & Supplies | M3C3 | 35.924 | 31.59 | 28.387 | 24.785 | 30.3 | 28.735 | 1.769 | 13.722 | 11.284 | 12.573 | 20.3075 | 2.30214 | 2.51569 | 1.97129 | 6.758 | 7.686 | 8.553 | 9.796 | 8.013 | 8.449 | NaN | NaN | NaN | NaN | NaN | NaN | 15.569 | 15.091 | 13.528 | 12.39 | 14.569 | 13.792 | 17.7305 | 1.336 | @:KKM3C3 | ||
Transportation | Transportation | M2TR | 53.824 | 27.13 | 21.459 | 18.841 | 24.86 | 22.217 | -37.762 | 98.395 | 26.795 | 13.592 | -1.42535 | 0.275725 | 0.800858 | 1.38618 | 9.784 | 19.411 | 24.541 | 27.951 | 21.183 | 23.703 | NaN | NaN | NaN | NaN | NaN | NaN | 17.145 | 13.568 | 11.881 | 11.194 | 12.902 | 12.114 | 26.8634 | 1.493 | @:KKM2TR | |
Air Freight & Couriers | M3AF | 23.481 | 18.544 | 17.43 | 16.622 | 18.362 | 17.807 | 23.379 | 26.624 | 6.391 | 4.861 | 1.00436 | 0.696514 | 2.72727 | 3.41946 | 13.206 | 16.721 | 17.79 | 18.655 | 16.887 | 17.413 | NaN | NaN | NaN | NaN | NaN | NaN | 11.507 | 10.768 | 10.292 | 10.096 | 10.643 | 10.401 | 43.3735 | 1.649 | @:KKM3AF | ||
Airlines | M3AL | NaN | NaN | 20.079 | 9.507 | NaN | 38.894 | -398.184 | NaN | NaN | 90.2 | NaN | NaN | NaN | 0.105399 | -44.106 | -15.7 | 7.046 | 14.88 | -6.955 | 3.637 | NaN | NaN | NaN | NaN | NaN | NaN | -10.761 | 36.019 | 6.961 | 5.249 | 13.894 | 7.918 | -7.2917 | 0.687 | @:KKM3AL | ||
Marine | M3MA | 22.851 | 11.016 | 14.868 | 13.704 | 12.057 | 14.049 | 172.952 | 107.444 | -25.911 | 8.493 | 0.132123 | 0.102528 | -0.57381 | 1.61356 | 21.273 | 44.131 | 32.696 | 35.473 | 40.319 | 34.602 | NaN | NaN | NaN | NaN | NaN | NaN | 26.332 | 5.73 | 7.333 | 6.751 | 6.176 | 7.002 | 31.5789 | 1.928 | @:KKM3MA | ||
Road & Rail | M3RR | 39.396 | 28.293 | 24.028 | 21.123 | 26.807 | 24.654 | 15.396 | 39.242 | 18.475 | 13.755 | 2.55885 | 0.720988 | 1.30057 | 1.53566 | 26.717 | 37.202 | 43.804 | 49.83 | 39.265 | 42.693 | NaN | NaN | NaN | NaN | NaN | NaN | 16.751 | 16.046 | 14.359 | 13.469 | 15.443 | 14.611 | 41.0569 | 1.433 | @:KKM3RR | ||
Transportation Infrastructure | M3TI | NaN | 662.062 | 49.975 | 30.378 | 102.955 | 51.555 | -146.422 | NaN | 1224.8 | 64.51 | NaN | NaN | 0.040803 | 0.470904 | -4.955 | 0.377 | 4.993 | 8.214 | 2.424 | 4.84 | NaN | NaN | NaN | NaN | NaN | NaN | 25.948 | 18.467 | 14.374 | 12.932 | 16.441 | 14.66 | -5.102 | 1.186 | @:KKM3TI | ||
Consumer Discretionary | Consumer Discretionary | Consumer Discretionary | M1CD | 58.928 | 33.173 | 25.395 | 21.756 | 30.087 | 26.429 | -36.663 | 77.638 | 30.626 | 17.235 | -1.60729 | 0.427278 | 0.829197 | 1.26232 | 7.852 | 13.949 | 18.221 | 21.269 | 15.379 | 17.508 | NaN | NaN | NaN | NaN | NaN | NaN | 18.65 | 15.512 | 12.861 | 12.701 | 14.517 | 13.245 | 15.6538 | 1.296 | @:KKM1CD |
Automobiles & Components | Automobiles & Components | M2AC | 51.593 | 19.646 | 15.871 | 14.223 | 18.203 | 16.396 | -53.414 | 162.616 | 23.783 | 11.586 | -0.96591 | 0.120812 | 0.667325 | 1.2276 | 6.271 | 16.467 | 20.384 | 22.745 | 17.773 | 19.731 | NaN | NaN | NaN | NaN | NaN | NaN | 10.494 | 7.109 | 6 | 6.017 | 6.705 | 6.165 | 12.4352 | 1.098 | @:KKM2AC | |
Auto Components | M3AU | 58.453 | 16.917 | 12.85 | 11.104 | 15.303 | 13.387 | -72.618 | 245.535 | 31.644 | 15.725 | -0.80494 | 0.068899 | 0.40608 | 0.706137 | 5.102 | 17.629 | 23.208 | 26.857 | 19.489 | 22.278 | NaN | NaN | NaN | NaN | NaN | NaN | 9.347 | 7.252 | 6.229 | 5.701 | 6.879 | 6.38 | 9.0395 | 1.305 | @:KKM3AU | ||
Automobiles | M3AM | 50.573 | 20.206 | 16.539 | 14.945 | 18.816 | 17.055 | -47.99 | 150.287 | 22.169 | 10.671 | -1.05382 | 0.134449 | 0.746042 | 1.40052 | 6.077 | 15.21 | 18.582 | 20.564 | 16.334 | 18.02 | NaN | NaN | NaN | NaN | NaN | NaN | 10.734 | 7.074 | 5.945 | 6.091 | 6.663 | 6.113 | 15.311 | 1.039 | @:KKM3AM | ||
Consumer Durables & Apparel | Consumer Durables & Apparel | M2CA | 39.496 | 24.894 | 21.827 | 19.543 | 23.978 | 22.45 | -17.331 | 58.657 | 14.053 | 12.841 | -2.27892 | 0.424399 | 1.55319 | 1.52192 | 10.379 | 16.467 | 18.781 | 20.975 | 17.096 | 18.259 | NaN | NaN | NaN | NaN | NaN | NaN | 17.608 | 14.899 | 13.523 | 20.34 | 14.542 | 13.896 | 24.3377 | 1.215 | @:KKM2CA | |
Household Durables | M3HD | 16.499 | 11.858 | 11.062 | 9.949 | 11.498 | 11.118 | 5.999 | 39.14 | 7.193 | 9.284 | 2.75029 | 0.302964 | 1.53788 | 1.07163 | 12.093 | 16.826 | 18.036 | 20.055 | 17.353 | 17.945 | NaN | NaN | NaN | NaN | NaN | NaN | 9.087 | 7.281 | 7.207 | 6.349 | 7.314 | 7.361 | 37.6744 | 2.044 | @:KKM3HD | ||
Leisure Equipment & Products | M3LE | 92.408 | 57.402 | 45.055 | 35.573 | 49.294 | 43.267 | -8.333 | 60.984 | 27.405 | 26.655 | -11.0894 | 0.941263 | 1.64404 | 1.33457 | 0.699 | 1.126 | 1.434 | 1.816 | 1.311 | 1.493 | NaN | NaN | NaN | NaN | NaN | NaN | 27.108 | 36.642 | 31.144 | 25.152 | 33.198 | 29.71 | 9.3023 | 2.776 | @:KKM3LE | ||
Textiles & Apparel | M3TA | 60.762 | 34.127 | 28.674 | 24.988 | 32.813 | 30.05 | -32.413 | 78.045 | 19.019 | 14.749 | -1.87462 | 0.437273 | 1.50765 | 1.69422 | 18.035 | 32.111 | 38.218 | 43.854 | 33.396 | 36.467 | NaN | NaN | NaN | NaN | NaN | NaN | 22.419 | 18.948 | 16.513 | 26.625 | 18.335 | 17.11 | 17.9191 | 0.995 | @:KKM3TA | ||
Hotels, Restaurants & Leisure | Hotels, Restaurants & Leisure | M2HR | NaN | 83.77 | 30.087 | 23.275 | 50.01 | 32.791 | -116.019 | NaN | 178.421 | 29.266 | NaN | NaN | 0.168629 | 0.795291 | -2.802 | 4.683 | 13.038 | 16.854 | 7.844 | 11.963 | NaN | NaN | NaN | NaN | NaN | NaN | 41.618 | 25.515 | 16.38 | 14.328 | 20.997 | 17.149 | 11.0818 | 1.122 | @:KKM2HR | |
Hotels Restaurants & Leisure | M3HR | NaN | 83.77 | 30.087 | 23.275 | 50.01 | 32.791 | -116.019 | NaN | 178.421 | 29.266 | NaN | NaN | 0.168629 | 0.795291 | -3.069 | 5.13 | 14.282 | 18.462 | 8.592 | 13.104 | NaN | NaN | NaN | NaN | NaN | NaN | 41.619 | 25.515 | 16.38 | 14.328 | 20.997 | 17.148 | 11.0818 | 1.122 | @:KKM3HR | ||
Media | Media | M2MD | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM2MD | |
Media | M3ME | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM3ME | ||
Retailing | Retailing | M2RT | 46.823 | 39.315 | 32.45 | 27.415 | 36.962 | 33.604 | 21.338 | 19.096 | 21.156 | 19.192 | 2.19435 | 2.05881 | 1.53384 | 1.42846 | 20.305 | 24.182 | 29.298 | 34.679 | 25.722 | 28.293 | NaN | NaN | NaN | NaN | NaN | NaN | 20.891 | 19.816 | 16.779 | 15.114 | 18.749 | 17.254 | 15.0235 | 1.578 | @:KKM2RT | |
Distributors | M3DI | 25.655 | 22.032 | 20.206 | 19.126 | 21.388 | 20.489 | 5.732 | 16.447 | 9.035 | 5.645 | 4.47575 | 1.33958 | 2.23641 | 3.38813 | 5.995 | 6.982 | 7.612 | 8.042 | 7.192 | 7.507 | NaN | NaN | NaN | NaN | NaN | NaN | 14.482 | 13.767 | 12.629 | NaN | 13.371 | 12.809 | 79.3103 | 1.626 | @:KKM3DI | ||
Internet & Catalog Retail | M3NT | 82.281 | 70.084 | 50.214 | 37.414 | 62.269 | 52.959 | 91.499 | 17.404 | 39.57 | 34.214 | 0.899256 | 4.02689 | 1.26899 | 1.09353 | 53.791 | 63.152 | 88.142 | 118.298 | 71.078 | 83.573 | NaN | NaN | NaN | NaN | NaN | NaN | 30.253 | 27.479 | 20.878 | 17.578 | 24.935 | 21.79 | -1.5748 | 1.155 | @:KKM3NT | ||
Multiline Retail | M3MR | 22.783 | 22.275 | 20.3 | 19.213 | 21.752 | 20.718 | 25.087 | 2.28 | 9.731 | 7.532 | 0.90816 | 9.76974 | 2.08612 | 2.55085 | 17.945 | 18.354 | 20.14 | 21.279 | 18.796 | 19.733 | NaN | NaN | NaN | NaN | NaN | NaN | 11.417 | 11.744 | 11.212 | 10.993 | 11.601 | 11.305 | 7.6923 | 1.453 | @:KKM3MR | ||
Specialty Retail | M3SR | 31.45 | 24.688 | 21.866 | 19.79 | 23.831 | 22.428 | -4.976 | 27.39 | 12.908 | 10.487 | -6.32034 | 0.901351 | 1.69399 | 1.8871 | 14.657 | 18.672 | 21.082 | 23.293 | 19.344 | 20.554 | NaN | NaN | NaN | NaN | NaN | NaN | 15.747 | 14.874 | 13.839 | 12.946 | 14.569 | 14.042 | 23.0047 | 1.64 | @:KKM3SR | ||
Consumer Staples | Consumer Staples | Consumer Staples | M1CS | 22.224 | 20.903 | 19.214 | 17.937 | 20.148 | 19.343 | -2.068 | 6.321 | 8.789 | 7.646 | -10.7466 | 3.30691 | 2.18614 | 2.34593 | 12.453 | 13.24 | 14.404 | 15.429 | 13.736 | 14.308 | NaN | NaN | NaN | NaN | NaN | NaN | 12.989 | 13.258 | 12.341 | 11.988 | 12.875 | 12.461 | 5.7784 | 2.653 | @:KKM1CS |
Food & Staples Retailing | Food & Staples Retailing | M2FD | 21.674 | 21.304 | 19.216 | 18.101 | 20.18 | 19.315 | 1.165 | 1.739 | 10.865 | 8.023 | 18.6043 | 12.2507 | 1.76861 | 2.25614 | 7.408 | 7.537 | 8.355 | 8.87 | 7.956 | 8.313 | NaN | NaN | NaN | NaN | NaN | NaN | 9.623 | 9.844 | 8.854 | 9.196 | 9.412 | 9.052 | 2.0772 | 1.943 | @:KKM2FD | |
Food & Staples Retailing | M3FD | 21.674 | 21.304 | 19.216 | 18.101 | 20.18 | 19.315 | 1.165 | 1.739 | 10.865 | 8.023 | 18.6043 | 12.2507 | 1.76861 | 2.25614 | 7.408 | 7.537 | 8.355 | 8.87 | 7.956 | 8.313 | NaN | NaN | NaN | NaN | NaN | NaN | 9.623 | 9.844 | 8.854 | 9.196 | 9.412 | 9.052 | 2.0772 | 1.943 | @:KKM3FD | ||
Food Beverage & Tobacco | Food Beverage & Tobacco | M2FB | 21.252 | 19.677 | 18.085 | 16.805 | 19.059 | 18.283 | -4.647 | 8 | 8.807 | 7.824 | -4.57327 | 2.45962 | 2.05348 | 2.14788 | 14.98 | 16.178 | 17.603 | 18.943 | 16.703 | 17.412 | NaN | NaN | NaN | NaN | NaN | NaN | 13.968 | 13.556 | 12.651 | 12.024 | 13.214 | 12.775 | 11.2885 | 2.988 | @:KKM2FB | |
Beverages | M3BV | 28.699 | 25.411 | 22.808 | 20.836 | 24.288 | 23.05 | -12.886 | 12.94 | 11.409 | 9.469 | -2.22715 | 1.96376 | 1.99912 | 2.20044 | 10.879 | 12.287 | 13.689 | 14.985 | 12.855 | 13.546 | NaN | NaN | NaN | NaN | NaN | NaN | 17.363 | 16.471 | 14.985 | 14.325 | 15.858 | 15.148 | 13.8667 | 2.268 | @:KKM3BV | ||
Food Products | M3FP | 23.178 | 21.801 | 20.185 | 19.155 | 21.209 | 20.418 | -2.089 | 6.313 | 8.008 | 6.433 | -11.0953 | 3.45335 | 2.5206 | 2.97762 | 13.16 | 13.991 | 15.111 | 15.924 | 14.382 | 14.938 | NaN | NaN | NaN | NaN | NaN | NaN | 14.535 | 13.866 | 13.12 | 12.759 | 13.607 | 13.246 | 7.7295 | 2.297 | @:KKM3FP | ||
Tobacco | M3TB | 11.53 | 10.933 | 10.2 | 9.459 | 10.673 | 10.307 | 1.092 | 5.464 | 7.183 | 7.831 | 10.5586 | 2.00092 | 1.42002 | 1.20789 | 31.013 | 32.707 | 35.056 | 37.801 | 33.501 | 34.693 | NaN | NaN | NaN | NaN | NaN | NaN | 9.075 | 9.434 | 8.923 | 8.336 | 9.254 | 8.998 | 17.0455 | 6.461 | @:KKM3TB | ||
Household & Personal Products | Household & Personal Products | M2HH | 25.369 | 24.013 | 22.438 | 21.001 | 23.167 | 22.35 | 2.737 | 5.647 | 7.019 | 6.841 | 9.26891 | 4.25235 | 3.19675 | 3.06987 | 12.939 | 13.67 | 14.63 | 15.63 | 14.169 | 14.687 | NaN | NaN | NaN | NaN | NaN | NaN | 14.612 | 16.74 | 15.872 | 15.13 | 16.299 | 15.865 | -8.1712 | 2.421 | @:KKM2HH | |
Household Products | M3HP | 23.577 | 22.34 | 20.964 | 19.697 | 21.553 | 20.821 | 7.146 | 5.54 | 6.563 | 6.434 | 3.29933 | 4.03249 | 3.19427 | 3.06139 | 12.993 | 13.712 | 14.612 | 15.552 | 14.213 | 14.713 | NaN | NaN | NaN | NaN | NaN | NaN | 15.414 | 15.735 | 15.096 | 14.314 | 15.428 | 15.034 | -23.5669 | 2.566 | @:KKM3HP | ||
Personal Products | M3PP | 29.354 | 27.722 | 25.661 | 23.82 | 26.746 | 25.726 | -5.876 | 5.887 | 8.03 | 7.73 | -4.99558 | 4.70902 | 3.19564 | 3.0815 | 13.384 | 14.172 | 15.31 | 16.494 | 14.689 | 15.272 | NaN | NaN | NaN | NaN | NaN | NaN | 12.985 | 18.664 | 17.33 | 16.657 | 17.951 | 17.432 | 16 | 2.162 | @:KKM3PP | ||
Health Care | Health Care | Health Care | M1HC | 20.773 | 18.076 | 16.795 | 15.617 | 17.652 | 17.003 | 5.684 | 14.924 | 7.628 | 7.26 | 3.65464 | 1.2112 | 2.20176 | 2.1511 | 15.82 | 18.181 | 19.568 | 21.044 | 18.618 | 19.328 | NaN | NaN | NaN | NaN | NaN | NaN | 14.201 | 13.127 | 12.059 | 11.091 | 12.766 | 12.225 | 9.2046 | 2.162 | @:KKM1HC |
Health Care Equipment & Services | Health Care Equipment & Services | M2HE | 26.702 | 22.633 | 20.717 | 18.617 | 22.057 | 21.075 | 5.235 | 17.977 | 9.246 | 10.781 | 5.10067 | 1.259 | 2.24064 | 1.72683 | 28.196 | 33.264 | 36.34 | 40.44 | 34.132 | 35.723 | NaN | NaN | NaN | NaN | NaN | NaN | 15.404 | 14.631 | 13.318 | 12.162 | 14.209 | 13.532 | 16.3949 | 1.215 | @:KKM2HE | |
Health Care Equipment & Supplies | M3HS | 40.8 | 30.91 | 28.421 | 25.64 | 30.267 | 28.973 | -2.203 | 31.996 | 8.759 | 10.518 | -18.5202 | 0.966058 | 3.24478 | 2.43773 | 17.679 | 23.335 | 25.379 | 28.132 | 23.831 | 24.896 | NaN | NaN | NaN | NaN | NaN | NaN | 24.441 | 20.84 | 18.854 | 17.352 | 20.268 | 19.238 | 19.0259 | 1.034 | @:KKM3HS | ||
Health Care Providers & Services | M3PS | 16.564 | 15.279 | 13.991 | 12.615 | 14.856 | 14.2 | 11.331 | 8.411 | 9.207 | 10.765 | 1.46183 | 1.81655 | 1.5196 | 1.17185 | 48.581 | 52.667 | 57.516 | 63.787 | 54.168 | 56.668 | NaN | NaN | NaN | NaN | NaN | NaN | 9.902 | 10.143 | 9.292 | 8.386 | 9.845 | 9.405 | 18.7831 | 1.446 | @:KKM3PS | ||
Pharmaceuticals, Biotechnology & Life Sciences | Pharmaceuticals, Biotechnology & Life Sciences | M2PB | 17.9 | 15.778 | 14.772 | 14.007 | 15.425 | 14.919 | 5.903 | 13.444 | 6.813 | 5.461 | 3.03236 | 1.17361 | 2.16821 | 2.56491 | 13.408 | 15.211 | 16.247 | 17.135 | 15.56 | 16.087 | NaN | NaN | NaN | NaN | NaN | NaN | 13.471 | 12.253 | 11.316 | 10.445 | 11.925 | 11.457 | 2.2046 | 2.814 | @:KKM2PB | |
Biotechnology | M3BI | 17.357 | 14.882 | 14.049 | 14.11 | 14.588 | 14.147 | 14.739 | 16.634 | 5.925 | -0.431 | 1.17762 | 0.894674 | 2.37114 | -32.7378 | 79.428 | 92.64 | 98.129 | 97.706 | 94.508 | 97.455 | NaN | NaN | NaN | NaN | NaN | NaN | 13.374 | 11.588 | 10.52 | 9.693 | 11.22 | 10.669 | -8.0729 | 3.459 | @:KKM3BI | ||
Pharmaceuticals | M3PH | 16.3 | 14.573 | 13.492 | 12.6 | 14.193 | 13.659 | 1.148 | 11.85 | 8.017 | 7.072 | 14.1986 | 1.22979 | 1.68292 | 1.78167 | 10.453 | 11.692 | 12.629 | 13.522 | 12.005 | 12.474 | NaN | NaN | NaN | NaN | NaN | NaN | 12.577 | 11.538 | 10.589 | 9.911 | 11.204 | 10.736 | -2.5591 | 3.029 | @:KKM3PH | ||
Financials | Financials | Financials | M1FN | 18.188 | 13.451 | 12.756 | 11.593 | 13.194 | 12.853 | -21.036 | 35.304 | 5.408 | 10.197 | -0.86461 | 0.381005 | 2.35873 | 1.1369 | 8.114 | 10.972 | 11.57 | 12.73 | 11.186 | 11.483 | NaN | NaN | NaN | NaN | NaN | NaN | 10.416 | 11.49 | 10.478 | 10.368 | 11.883 | 11.666 | 22.0286 | 2.308 | @:KKM1FN |
Banks | Banks | M2B2 | 17.68 | 11.913 | 11.521 | 10.397 | 11.756 | 11.561 | -34.349 | 48.413 | 3.399 | 10.811 | -0.51472 | 0.24607 | 3.38953 | 0.961706 | 6.262 | 9.293 | 9.609 | 10.648 | 9.417 | 9.576 | NaN | NaN | NaN | NaN | NaN | NaN | 6.124 | 17.066 | 5.678 | 5.159 | 16.844 | 16.52 | 30.6991 | 2.347 | @:KKM2B2 | |
M3B3 | 17.68 | 11.913 | 11.521 | 10.397 | 11.756 | 11.561 | -34.349 | 48.413 | 3.399 | 10.811 | -0.51472 | 0.24607 | 3.38953 | 0.961706 | 7.029 | 10.432 | 10.787 | 11.953 | 10.572 | 10.75 | NaN | NaN | NaN | NaN | NaN | NaN | 6.124 | 17.066 | 5.678 | 5.159 | 16.843 | 16.518 | 30.6991 | 2.347 | @:KKM3B3 | |||
Diversified Financials | Diversified Financials | M2D2 | 21.441 | 16.666 | 15.937 | 14.515 | 16.421 | 16.058 | 0.779 | 28.861 | 4.544 | 9.921 | 27.5237 | 0.577457 | 3.50726 | 1.46306 | 9.937 | 12.785 | 13.369 | 14.679 | 12.975 | 13.269 | NaN | NaN | NaN | NaN | NaN | NaN | 13.125 | 11.68 | 12.407 | 12.471 | 12.45 | 12.366 | 27.7835 | 1.743 | @:KKM2D2 | |
Diversified Financial Services | M3D3 | 22.08 | 22.003 | 20.207 | 19.422 | 21.441 | 20.502 | -5.555 | 2.518 | 9.449 | 5.221 | -3.9748 | 8.73828 | 2.13853 | 3.71998 | 8.472 | 8.501 | 9.257 | 9.632 | 8.725 | 9.124 | NaN | NaN | NaN | NaN | NaN | NaN | 4.171 | 12.159 | 18.297 | 22.226 | 13.454 | 16.02 | 2.7778 | 2.205 | @:KKM3D3 | ||
Insurance | Insurance | M2I2 | 15.604 | 13.018 | 11.77 | 10.817 | 12.563 | 11.956 | -10.837 | 19.865 | 10.603 | 9.244 | -1.43988 | 0.655323 | 1.11006 | 1.17016 | 8.936 | 10.712 | 11.847 | 12.891 | 11.1 | 11.664 | NaN | NaN | NaN | NaN | NaN | NaN | 6.659 | 10.74 | 8.204 | 8.027 | 10.054 | 9.496 | 6.3123 | 2.946 | @:KKM2I2 | |
Insurance | M3I3 | 15.604 | 13.018 | 11.77 | 10.817 | 12.563 | 11.956 | -10.837 | 19.865 | 10.603 | 9.244 | -1.43988 | 0.655323 | 1.11006 | 1.17016 | 8.936 | 10.712 | 11.847 | 12.891 | 11.1 | 11.664 | NaN | NaN | NaN | NaN | NaN | NaN | 6.659 | 10.74 | 8.204 | 8.027 | 10.054 | 9.496 | 6.3123 | 2.946 | @:KKM3I3 | ||
Real Estate | Real Estate | Real Estate | M1RE | 36.133 | 33.914 | 30.25 | 27.612 | 32.437 | 30.644 | -21.715 | 6.544 | 12.112 | 10.911 | -1.66397 | 5.18246 | 2.49752 | 2.53066 | 32.691 | 34.831 | 39.049 | 42.78 | 36.417 | 38.547 | NaN | NaN | NaN | NaN | NaN | NaN | 20.855 | 20.721 | 19.281 | 18.252 | 20.155 | 19.459 | 5.9977 | 2.843 | @:KKM1RE |
Information Technology | Information Technology | Information Technology | M1IT | 37.748 | 30.039 | 26.845 | 24.37 | 28.335 | 26.841 | 6.814 | 25.662 | 11.9 | 10.227 | 5.53977 | 1.17056 | 2.25588 | 2.38291 | 13.597 | 17.086 | 19.12 | 21.061 | 18.114 | 19.122 | NaN | NaN | NaN | NaN | NaN | NaN | 20.718 | 19.898 | 18.211 | 16.735 | 18.997 | 18.15 | 15.8019 | 1.057 | @:KKM1IT |
Software & Services | Software & Services | M2SS | 45.52 | 38.507 | 33.759 | 29.245 | 36.136 | 33.62 | 6.832 | 18.212 | 14.065 | 15.399 | 6.66276 | 2.11438 | 2.40021 | 1.89915 | 12.943 | 15.3 | 17.452 | 20.145 | 16.304 | 17.524 | NaN | NaN | NaN | NaN | NaN | NaN | 25.324 | 25.142 | 22.474 | 20.2 | 23.642 | 22.238 | 7.9109 | 1.047 | @:KKM2SS | |
Internet Software & Services | M3NS | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | @:KKM3NS | ||
IT Consulting & Services | M3IS | 45.992 | 38.853 | 32.506 | 27.986 | 35.945 | 32.975 | -10.357 | 18.375 | 19.524 | 16.287 | -4.44067 | 2.11445 | 1.66493 | 1.7183 | 5.591 | 6.619 | 7.911 | 9.188 | 7.154 | 7.798 | NaN | NaN | NaN | NaN | NaN | NaN | 24.436 | 24.713 | 21.993 | 19.288 | 23.406 | 22.188 | 5.2567 | 1.195 | @:KKM3IS | ||
Software | M3SW | 45.212 | 38.281 | 34.643 | 30.135 | 36.264 | 34.063 | 22.094 | 18.105 | 10.501 | 14.779 | 2.04635 | 2.11439 | 3.29902 | 2.03904 | 15.781 | 18.639 | 20.596 | 23.677 | 19.675 | 20.947 | NaN | NaN | NaN | NaN | NaN | NaN | 26.156 | 25.777 | 23.112 | 21.106 | 24.093 | 22.568 | 10.1331 | 0.956 | @:KKM3SW | ||
Technology Hardware & Equipment | Technology Hardware & Equipment | M2TH | 32.33 | 24.02 | 22.481 | 21.405 | 23.122 | 22.447 | 6.127 | 34.594 | 6.846 | 5.092 | 5.27664 | 0.69434 | 3.28382 | 4.20365 | 15.139 | 20.377 | 21.772 | 22.866 | 21.169 | 21.805 | NaN | NaN | NaN | NaN | NaN | NaN | 17.952 | 15.71 | 15.234 | 14.301 | 15.477 | 15.217 | 25.4369 | 0.916 | @:KKM2TH | |
Communications Equipment | M3CE | 17.311 | 17.305 | 15.71 | 14.667 | 16.446 | 15.718 | 5.552 | 0.039 | 10.154 | 7.105 | 3.11798 | 443.718 | 1.54717 | 2.06432 | 5.03 | 5.032 | 5.543 | 5.937 | 5.295 | 5.54 | NaN | NaN | NaN | NaN | NaN | NaN | 9.622 | 10.848 | 9.912 | 9.363 | 10.398 | 9.973 | 14.8649 | 2.394 | @:KKM3CE | ||
Computers & Peripherals | M3CP | 36.664 | 25.09 | 23.742 | 22.894 | 24.225 | 23.66 | 8.251 | 46.13 | 5.676 | 4.095 | 4.44358 | 0.543898 | 4.18288 | 5.59072 | 39.11 | 57.151 | 60.395 | 62.632 | 59.191 | 60.606 | NaN | NaN | NaN | NaN | NaN | NaN | 20.696 | 16.842 | 16.623 | 15.683 | 16.705 | 16.562 | 30.5263 | 0.695 | @:KKM3CP | ||
Electrical Equipment | M3EE | 39.488 | 29.788 | 25.659 | 22.997 | 27.976 | 26.015 | -21.317 | 32.564 | 16.091 | 11.573 | -1.85242 | 0.914752 | 1.59462 | 1.98713 | 12.121 | 16.069 | 18.654 | 20.813 | 17.109 | 18.399 | NaN | NaN | NaN | NaN | NaN | NaN | 18.721 | 17.625 | 15.216 | 14.024 | 16.62 | 15.433 | 21.0191 | 1.802 | @:KKM3EE | ||
Semiconductors & Semiconductor Equipment | M3SC | 30.655 | 23.983 | 20.864 | 19.278 | 22.228 | 21.033 | 7.648 | 27.819 | 14.952 | 8.012 | 4.00824 | 0.862109 | 1.3954 | 2.40614 | 24.361 | 31.138 | 35.794 | 38.738 | 33.597 | 35.506 | NaN | NaN | NaN | NaN | NaN | NaN | 15.988 | 16.402 | 14.498 | 13.561 | 15.426 | 14.597 | 29.7872 | 1.276 | @:KKM3SC | ||
Telecommunication Services | Telecommunication Services | Telecommunication Services | M1T1 | 28.425 | 23.756 | 20.627 | 17.992 | 22.492 | 21.005 | -1.625 | 19.657 | 15.171 | 14.691 | -17.4923 | 1.20853 | 1.35963 | 1.2247 | 3.402 | 4.071 | 4.688 | 5.375 | 4.299 | 4.604 | NaN | NaN | NaN | NaN | NaN | NaN | 11.184 | 11.288 | 9.766 | 9.564 | 10.704 | 9.97 | 10.5736 | 3.021 | @:KKM1T1 |
M2T2 | 13.524 | 13.04 | 12.425 | 11.494 | 12.861 | 12.552 | -5.337 | 3.716 | 4.944 | 7.867 | -2.53401 | 3.50915 | 2.51315 | 1.46104 | 4.423 | 4.587 | 4.814 | 5.205 | 4.651 | 4.766 | NaN | NaN | NaN | NaN | NaN | NaN | 6.605 | 6.709 | 6.655 | 6.245 | 6.7 | 6.673 | 0.7092 | 5.202 | @:KKM2T2 | |||
Diversified Telecommunications Services | M3DT | 12.326 | 11.976 | 11.565 | 10.959 | 11.844 | 11.636 | -5.364 | 2.917 | 3.558 | 5.12 | -2.29791 | 4.10559 | 3.25042 | 2.14043 | 4.117 | 4.237 | 4.388 | 4.63 | 4.284 | 4.361 | NaN | NaN | NaN | NaN | NaN | NaN | 6.442 | 6.606 | 6.565 | 6.184 | 6.595 | 6.574 | -0.6148 | 5.195 | @:KKM3DT | ||
Wireless Telecommunication Services | M3WT | 27.564 | 24.378 | 20.363 | 15.335 | 23.369 | 21.351 | -5.017 | 13.069 | 19.721 | 32.786 | -5.49412 | 1.86533 | 1.03255 | 0.46773 | 3.953 | 4.47 | 5.351 | 7.105 | 4.663 | 5.103 | NaN | NaN | NaN | NaN | NaN | NaN | 7.615 | 7.321 | 7.186 | 6.588 | 7.327 | 7.259 | 9.2105 | 5.277 | @:KKM3WT | ||
Utilities | Utilities | Utilities | M1U1 | 19.71 | 18.857 | 17.477 | 16.657 | 18.396 | 17.707 | -2.387 | 4.523 | 7.892 | 4.925 | -8.25723 | 4.16914 | 2.21452 | 3.38213 | 8.694 | 9.087 | 9.804 | 10.287 | 9.315 | 9.677 | NaN | NaN | NaN | NaN | NaN | NaN | 11.258 | 10.9 | 10.544 | 10.382 | 10.765 | 10.587 | 1.2915 | 3.526 | @:KKM1U1 |
M2U2 | 19.71 | 18.857 | 17.477 | 16.657 | 18.396 | 17.707 | -2.387 | 4.523 | 7.892 | 4.925 | -8.25723 | 4.16914 | 2.21452 | 3.38213 | 8.694 | 9.087 | 9.804 | 10.287 | 9.315 | 9.677 | NaN | NaN | NaN | NaN | NaN | NaN | 11.258 | 10.9 | 10.544 | 10.382 | 10.765 | 10.587 | 1.2915 | 3.526 | @:KKM2U2 | |||
Electric Utilities | M3EU | 19.302 | 18.744 | 17.591 | 16.734 | 18.346 | 17.776 | -2.881 | 2.978 | 6.556 | 5.117 | -6.69976 | 6.29416 | 2.68319 | 3.27028 | 11.598 | 11.943 | 12.726 | 13.377 | 12.202 | 12.593 | NaN | NaN | NaN | NaN | NaN | NaN | 11.176 | 11.036 | 10.71 | 10.517 | 10.924 | 10.763 | -2.1544 | 3.527 | @:KKM3EU | ||
Gas Utilities | M3GU | 20.194 | 18.64 | 17.729 | 17.078 | 18.12 | 17.708 | -1.761 | 8.335 | 5.142 | 3.808 | -11.4673 | 2.23635 | 3.44788 | 4.48477 | 14.695 | 15.92 | 16.739 | 17.376 | 16.377 | 16.758 | NaN | NaN | NaN | NaN | NaN | NaN | 11.167 | 11.171 | 10.845 | 10.579 | 10.987 | 10.84 | 6.7308 | 4.292 | @:KKM3GU | ||
Multi-Utilities | M3MU | 19.812 | 17.869 | 16.638 | 15.828 | 17.518 | 16.878 | -0.234 | 10.874 | 7.398 | 5.115 | -84.6667 | 1.64328 | 2.24899 | 3.09443 | 3.01 | 3.337 | 3.584 | 3.767 | 3.404 | 3.533 | NaN | NaN | NaN | NaN | NaN | NaN | 11.306 | 10.295 | 10.087 | 9.898 | 10.186 | 10.077 | 5.414 | 3.583 | @:KKM3MU | ||
Water Utilities | M3WU | 29.442 | 27.446 | 24.938 | 24.373 | 26.912 | 25.648 | -8.524 | 7.273 | 10.057 | 2.319 | -3.45401 | 3.77368 | 2.47967 | 10.5101 | 16.443 | 17.639 | 19.413 | 19.863 | 17.989 | 18.876 | NaN | NaN | NaN | NaN | NaN | NaN | 17.429 | 15.54 | 14.781 | 14.904 | 15.375 | 14.994 | 8.3333 | 2.455 | @:KKM3WU |
Conclusion
One can easily use interactive widgets to intuitively get dsws IBES GA data using our Python Get_IBES_GA function created above. We can even generate excel sheets replicating the 'Datastream IBES Global Aggregate MSCI.xlsm' file. However, this is only Part 1; I will attempt to see the best ways in which the above can be used to extract insights, possibly in graphical ways. If you have a workflow in mind for which the above would be useful, I would be happy to hear about it. Please do not hesitate to submit your propositions to jonathan.legrand@refinitiv.com