Author:
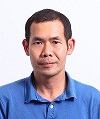
Simple Object Access Protocol (SOAP) was initially developed by Microsoft in 1998. It is a lightweight XML-based protocol for exchanging structured information over various standard network protocols, such as Hypertext Transfer Protocol (HTTP). It is typically used to implement web services. SOAP also provides Web Services Description Language (WSDL) which is an XML based definition language. The file is used for describing the functionality of a SOAP based web service. There are several tools that can parse WSDL files and then generate classes or libraries in different programing languages, such as C#, Python, and Java to access SOAP based web services.
This article introduces the wsdl2java tool in Apache CXF which is an open-source tool that can be used to generate JAVA classes and libraries from WSDL files. It also demonstrates how to use the wsdl2java tool on Maven to generate a Java SOAP client library from a DACS Station web service. To use Apache CXF with Eclipse, please refer to the How to use a Java SOAP client library created from wsdl2java and Apache CXF on Eclipse article.
Apache CXF
Apache CXF is an open source software project. It helps developers build and develop Web services frameworks that support a variety of protocols such as SOAP, XML/HTTP, RESTful HTTP, or CORBA. It also provides tools for generating code, generating WSDLs, adding endpoints and support files, and validating WSDLs. The following sections will demonstrate how to use the wsdl2java tool in Apache CXF to generate a Java SOAP client library from a SOAP web service (DACS Station Web Service) on Maven.
Setup an environment
The versions of Software applications used in this article are:
- Java 17.0.9
- Apache CXF 4.0.3
- Apache Maven 3.9.6
Download and install the Java and Apache Maven applications on the machine. Then, set the following environment variables.
Variable Name | Value | Example |
---|---|---|
JAVA_HOME | <Java installation path> | C:\Program Files\Java\jdk-17 |
PATH | <Java installation path>/bin <Maven installation path>/bin |
C:\Program Files\Java\jdk-17; C:\tools \apache-maven-3.9.6\bin |
On Windows operating systems, the following commands can be used.
SET JAVA_HOME=C:\Program Files\Java\jdk-17
SET PATH=%JAVA_HOME%\bin;C:\tools\apache-maven-3.9.6\bin;%PATH%
On Linux operating systems, the following commands can be used.
export JAVA_HOME=/usr/java/jdk1.7.0_05
export PATH=$JAVA_HOME/bin:/opt/tools/apache-maven-3.9.6/bin:$PATH
Run the following commands to verify the environment variables are set correctly.
C:\>java --version
java 17.0.9 2023-10-17 LTS
Java(TM) SE Runtime Environment (build 17.0.9+11-LTS-201)
Java HotSpot(TM) 64-Bit Server VM (build 17.0.9+11-LTS-201, mixed mode, sharing)
C:\>mvn --version
Apache Maven 3.9.6 (bc0240f3c744dd6b6ec2920b3cd08dcc295161ae)
Maven home: C:\D_DRIVE\apache-maven-3.9.6
Java version: 17.0.9, vendor: Oracle Corporation, runtime: C:\Program Files\Java\jdk-17
Default locale: en_US, platform encoding: UTF-8
OS name: "windows 10", version: "10.0", arch: "amd64", family: "windows"
Note: Apache CXF will be loaded by Maven while building Java projects.
Use wsdl2java and Apache CXF on Maven
wsdl2java is a tool in the Apache CXF that takes a WSDL document and generates fully annotated Java code or library from which to implement a service. In this section, I will demonstrate how to use this tool to generate a Java library for the DACS Station Web Service and then use this library on Maven.
1. Generate a Java project
Run the following Maven command to generate a Java project
mvn archetype:generate -DgroupId=com.lseg.dacsstation -DartifactId=dacs-client -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command will generate the Java project with the following structure. The pom.xml is in the dacs-client folder.

The source code file (App.java) is in the dacs-client\src\main\java\com\lseg\dacsstation folder.

The package name in the source code is com.lseg.dacstation and Maven also generates the sample “Hello World” code.
package com.lseg.dacsstation;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args )
{
System.out.println( "Hello World!" );
}
}
2. Add Apache CXF libraries as dependencies
Apache CXF libraries are available in the Maven Repository.
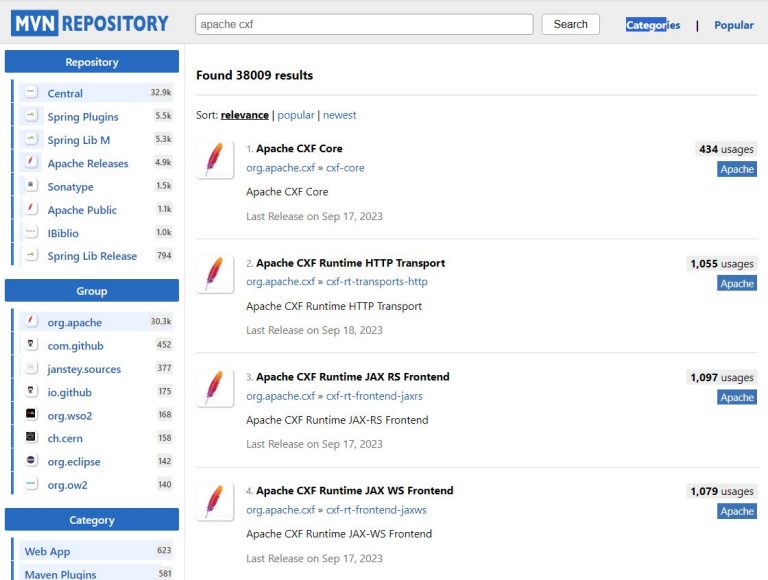
Add the following Apache CXF libraries to the dependencies section in the pom.xml file of the Java project.
The content in the pom.xml file looks like this:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
…
<properties>
<cxf.version>4.0.3</cxf.version>
</properties>
<dependencies>
<dependency>
…
<!-- https://mvnrepository.com/artifact/org.apache.cxf/cxf-rt-frontend-jaxws -->
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>${cxf.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.cxf/cxf-rt-frontend-jaxrs -->
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxrs</artifactId>
<version>${cxf.version}</version>
</dependency>
</dependencies>
….
</projects>
The version of Apache CXF is defined in the cxf.version property and it is used to specify the version of the Apache CXF libraries in the dependencies section. The version of Apache CXF is 4.0.3.
3. Add the Apache CXF plugin used to generate code
The Apache CXF provides the cxf-codegen-plugin plugin that can run the wsdl2java tool to generate Java code from WSDL files. Add the following plugin to the build section in the pom.xml of the Java project.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
…
<build>
<plugins>
<plugin>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-codegen-plugin</artifactId>
<version>${cxf.version}</version>
<executions>
<execution>
<id>generate-sources</id>
<phase>generate-sources</phase>
<configuration>
<sourceRoot>${project.build.directory}/generated-sources/cxf</sourceRoot>
<wsdlOptions>
<wsdlOption>
<wsdl>http://<DACS IP Address>:8080/DacsWS/DacsWebServiceService?wsdl</wsdl>
</wsdlOption>
</wsdlOptions>
</configuration>
<goals>
<goal>wsdl2java</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
….
</projects>
The version of the plugin is specified in the cxf.version property. It sets the goal element to the wsdl2java tool and sets the WSDL URL in the wsdlOption element. For example, http://<DACS_IP_Address>:8080/DacsWS/DacsWebServiceService?wsdl is the location of the WSDL file of the DACS Station Web Service.
The complete pom.xml file is available on GitHub.
4. Add the DACS client sample code
The source code file is App.java which is in the dacs-client\src\main\java\com\lseg\dacsstation folder. The source code is available on GitHub.
In the source code, change the <DACS IP Address> in the dacs_wsdl variable to the IP address of a DACS server and change the <DACS Admin> and <DACS Admin Password> in the dacs_username, and dacs_password variables respectively.
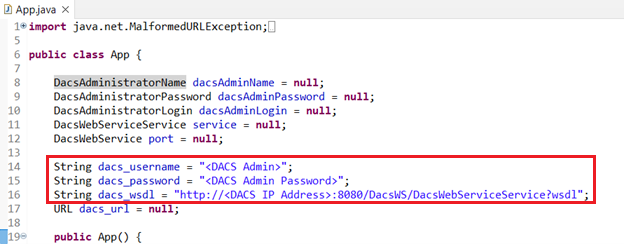
The example connects to the DACS Station Web Service and then displays DACS version, DACS site names, and DACS users, and entitlement data.
5. Compile and run the example
Then, run the following mvn command to compile the example.
mvn clean compile
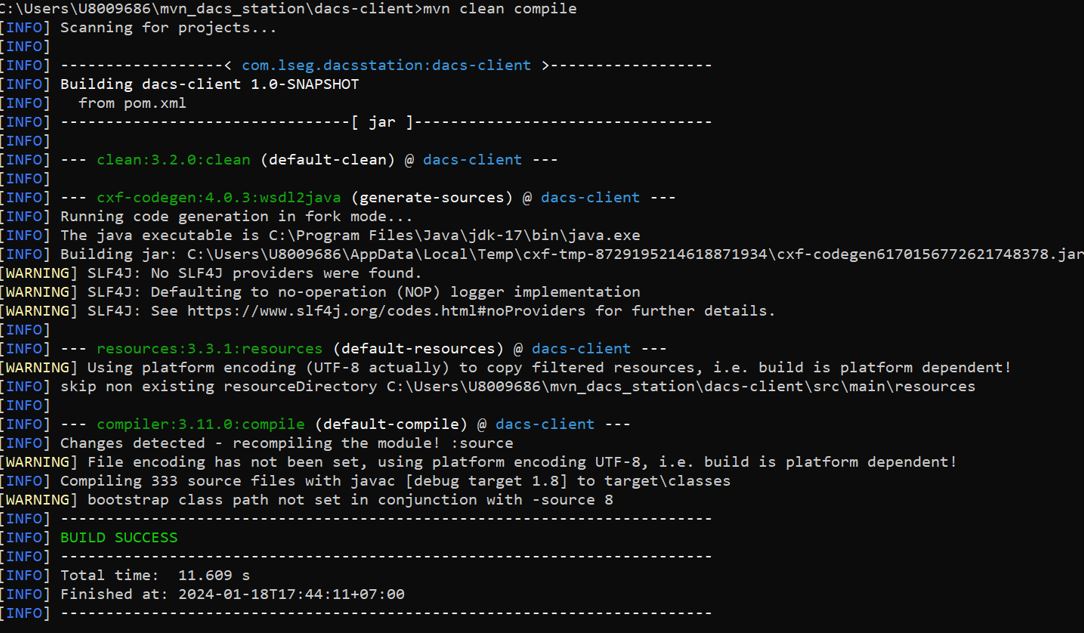
If it doesn’t have any errors, run the following mvn command to run the example.
mvn exec:java -Dexec.mainClass=com.lseg.dacsstation.App
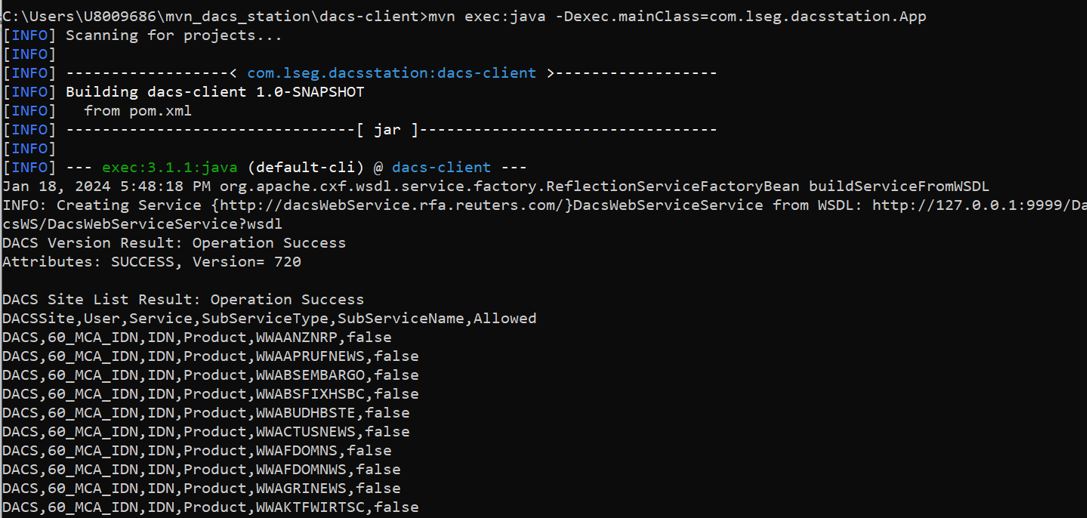
The example also creates the dacs_permission.csv file that contains entitlement data for all users in DACS.
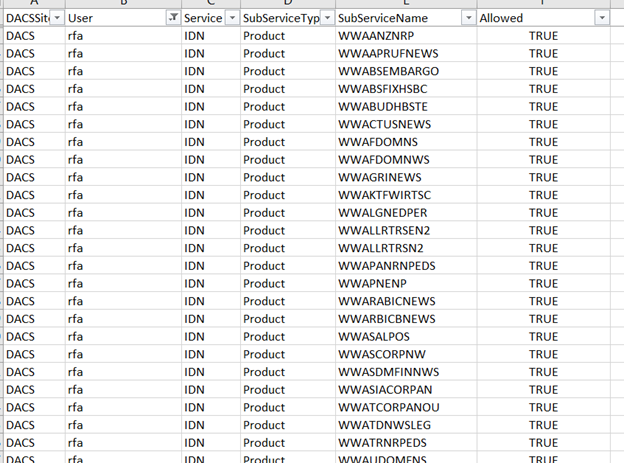
Summary
Simple Object Access Protocol (SOAP) is an XML-based web service protocol. Each SOAP web service provides a WSDL file which describes the definition and functionality of the web service. Wsdl2java is a tool in the Apache CXF that can generate Java classes and libraries from WSDL files. The Apache CXF libraries and wsdl2java tool can be added to the dependencies and plugins sections in the pom.xml file of the Maven Java project. Then, Maven will download the Apache CXF libraries and run the wsdl2java tool to generate SOAP client Java classes from a WSDL file. Finally, the generated Java classes can be used in the Maven Java project source files.
References
- Apache CXF: An open-source services framework (no date) Apache CXF -- Index. Available at: https://cxf.apache.org/ (Accessed: 08 January 2024).
- Gillis, A.S. (2022) What is soap?, App Architecture. Available at: https://www.techtarget.com/searchapparchitecture/definition/SOAP-Simple-Object-Access-Protocol (Accessed: 08 January 2024).
- Porter, B., Zyl, J. van and Lamy, O. (no date) Welcome to Apache Maven, Maven. Available at: https://maven.apache.org/ (Accessed: 08 January 2024).
- Soap (2023) Wikipedia. Available at: https://en.wikipedia.org/wiki/SOAP (Accessed: 08 January 2024).