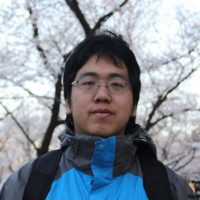
This article will cover how to use the Refinitiv Workspace Web Side by Side Integration API to exchange context with a web application on the same browser.
Sending context to Refinitiv Workspace Web:
Receiving context from Refinitiv Workspace Web:
Prerequisite:
- Refinitiv Workspace Web running on Chrome Browser
- Web server, to host the sample web app
Here is the sample web app code which demonstrate context sending/receiving API call.
Please create an index.html (or any preferred name) on your web server.
Then, you can add this code to the file.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>SxS Test Web App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this sample</noscript>
<script async src="https://cdn.refinitiv.com/public/libs/sxs-web/sxs-web-loader.js"></script>
<script>
function sendContext(ric){
//context, channelID
//sxsWeb.setContext({_context: {entities: [{RIC: ric}]}},1);
sxsWeb.setContext(sxsWeb.Context.ric(ric),1);
};
function contextReceived(contextData){
contextText = JSON.stringify(contextData._context, undefined, 2);
document.getElementById("outputArea").value = contextText;
}
window.SxsWeb = window.SxsWeb || [];
window.sxsWeb = window.sxsWeb || function () {
SxsWeb.push(arguments);
};
function registerOnContextChange(){
//register callback function
sxsWeb.onContextChange(contextReceived);
};
// Uncomment next line if you are using private network
//sxsWeb ("config", "use-network", "private");
sxsWeb('config','context','both');//none, both, send, receive
sxsWeb("start", new Date());
sxsWeb("onLoad", (status) => {
if (status === 'ok') {
console.log("Sxs Web is loaded");
//enable test buttons
document.getElementById("ibmContextButton").removeAttribute("disabled");
document.getElementById("ibmContextButton").value = "send IBM.N context to Workspace Web";
document.getElementById("pttContextButton").removeAttribute("disabled");
document.getElementById("pttContextButton").value = "send PTT.BK context to Workspace Web";
document.getElementById("registerOnContextChange").removeAttribute("disabled");
}
else {
console.log("Some issue detected", status);
}
});
</script>
Sending Context:<br/><br/>
<input type='button' id='ibmContextButton' disabled value='Not ready'
onclick='sendContext("IBM.N")'>
<br/><br/>
<input type='button' id='pttContextButton' disabled value='Not ready'
onclick='sendContext("PTT.BK")'>
<br/><br/><br/><br/>
Receiving Context:<br/><br/>
<input type='button' id='registerOnContextChange' disabled
value='Register On Context Change Event' onclick='registerOnContextChange()'>
<br/><br/>
<textarea rows="10" cols="50" id="outputArea"></textarea>
</body>
</html>
To test the application, simple launch Refinitiv Workspace Web on Chrome browser.
Then launch the sample web app on the same Chrome browser.
You can click (1) button to simulate context send function.
You can click (2) button to simulate context receive function.
Explaination on the sample code and API calls.
Step 1. The script is downloaded from Refinitiv CDN
<script async src="https://cdn.refinitiv.com/public/libs/sxs-web/sxs-web-loader.js"></script>
Step 2. Initialize the API.
sxsWeb('config','context','both');
sxsWeb("start", new Date());
sxsWeb("onLoad", (status) => {
...
...
...
Step 3. Send a context with sxsWeb.setContext() API call
sxsWeb.setContext(sxsWeb.Context.ric("A_VALID_RIC"),1);
Step 4. Register a callback function to sxsWeb.onContextChange() to listen to the onContextChange event.
sxsWeb.onContextChange(contextReceived);
//contextReceived is a function defined in this script, see Step 5.
Step 5. Implement callback function, how to process the onContextChange event
function contextReceived(contextData){
contextText = JSON.stringify(contextData._context, undefined, 2);
//Context in JSON format is available from contextData._context
...
...
...
};